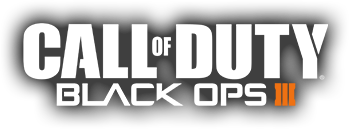 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\codescripts\struct;
3 #using scripts\shared\array_shared;
4 #using scripts\shared\callbacks_shared;
5 #using scripts\shared\demo_shared;
6 #using scripts\shared\laststand_shared;
7 #using scripts\shared\util_shared;
9 #using scripts\shared\ai\zombie_utility;
11 #insert scripts\shared\shared.gsh;
13 #using scripts\zm\_util;
14 #using scripts\zm\_zm;
15 #using scripts\zm\_zm_audio;
16 #using scripts\zm\_zm_laststand;
17 #using scripts\zm\_zm_lightning_chain;
18 #using scripts\zm\_zm_net;
19 #using scripts\zm\_zm_score;
20 #using scripts\zm\_zm_spawner;
21 #using scripts\zm\_zm_utility;
22 #using scripts\zm\_zm_weap_tesla;
23 #using scripts\zm\_zm_weapons;
25 #precache( "fx", "zombie/fx_tesla_rail_view_zmb" );
26 #precache( "fx", "zombie/fx_tesla_tube_view_zmb" );
27 #precache( "fx", "zombie/fx_tesla_tube_view2_zmb" );
28 #precache( "fx", "zombie/fx_tesla_tube_view3_zmb" );
29 #precache( "fx", "zombie/fx_tesla_rail_view_ug_zmb" );
30 #precache( "fx", "zombie/fx_tesla_tube_view_ug_zmb" );
31 #precache( "fx", "zombie/fx_tesla_tube_view2_ug_zmb" );
32 #precache( "fx", "zombie/fx_tesla_tube_view3_ug_zmb" );
41 level.weaponZMTeslaGun = GetWeapon(
"tesla_gun" );
42 level.weaponZMTeslaGunUpgraded = GetWeapon(
"tesla_gun_upgraded" );
48 level._effect[
"tesla_viewmodel_rail"] =
"zombie/fx_tesla_rail_view_zmb";
49 level._effect[
"tesla_viewmodel_tube"] =
"zombie/fx_tesla_tube_view_zmb";
50 level._effect[
"tesla_viewmodel_tube2"] =
"zombie/fx_tesla_tube_view2_zmb";
51 level._effect[
"tesla_viewmodel_tube3"] =
"zombie/fx_tesla_tube_view3_zmb";
52 level._effect[
"tesla_viewmodel_rail_upgraded"] =
"zombie/fx_tesla_rail_view_ug_zmb";
53 level._effect[
"tesla_viewmodel_tube_upgraded"] =
"zombie/fx_tesla_tube_view_ug_zmb";
54 level._effect[
"tesla_viewmodel_tube2_upgraded"] =
"zombie/fx_tesla_tube_view2_ug_zmb";
55 level._effect[
"tesla_viewmodel_tube3_upgraded"] =
"zombie/fx_tesla_tube_view3_ug_zmb";
57 level._effect[
"tesla_shock_eyes"] =
"zombie/fx_tesla_shock_eyes_zmb";
73 level.zombie_vars[
"tesla_max_enemies_killed"],
74 level.zombie_vars[
"tesla_radius_start"],
75 level.zombie_vars[
"tesla_radius_decay"],
76 level.zombie_vars[
"tesla_head_gib_chance"],
77 level.zombie_vars[
"tesla_arc_travel_time"],
78 level.zombie_vars[
"tesla_kills_for_powerup"],
79 level.zombie_vars[
"tesla_min_fx_distance"],
80 level.zombie_vars[
"tesla_network_death_choke"],
92 player endon(
"disconnect" );
94 if (
IS_TRUE( player.tesla_firing ) )
100 if( IsDefined(
self.zombie_tesla_hit ) &&
self.zombie_tesla_hit )
110 player.tesla_enemies = undefined;
111 player.tesla_enemies_hit = 1;
112 player.tesla_powerup_dropped =
false;
113 player.tesla_arc_count = 0;
114 player.tesla_firing = 1;
118 if( player.tesla_enemies_hit >= 4)
123 player.tesla_enemies_hit = 0;
124 player.tesla_firing = 0;
129 return ( (weapon == level.weaponZMTeslaGun || weapon == level.weaponZMTeslaGunUpgraded ) && (mod ==
"MOD_PROJECTILE" || mod ==
"MOD_PROJECTILE_SPLASH" ));
134 return IS_TRUE(
self.tesla_death );
148 self endon(
"disconnect" );
159 if( (
result ==
"weapon_change" ||
result ==
"grenade_fire" ) && (
self GetCurrentWeapon() == level.weaponZMTeslaGun ||
self GetCurrentWeapon() == level.weaponZMTeslaGunUpgraded) )
161 if(!IsDefined (
self.tesla_loop_sound))
163 self.tesla_loop_sound =
spawn(
"script_origin",
self.origin);
164 self.tesla_loop_sound linkto(
self);
167 self.tesla_loop_sound PlayLoopSound(
"wpn_tesla_idle", 0.25 );
173 self notify (
"weap_away");
174 if(IsDefined (
self.tesla_loop_sound))
176 self.tesla_loop_sound StopLoopSound(0.25);
184 self waittill(
"disconnect" );
193 self endon(
"disconnect" );
194 self endon (
"weap_away");
197 wait(randomintrange(7,15));
204 self endon(
"disconnect" );
205 self endon(
"death" );
209 self waittill(
"weapon_pvp_attack", attacker, weapon,
damage, mod );
216 if ( weapon != level.weaponZMTeslaGun && weapon != level.weaponZMTeslaGunUpgraded )
221 if ( mod !=
"MOD_PROJECTILE" && mod !=
"MOD_PROJECTILE_SPLASH" )
226 if (
self == attacker )
228 damage = int(
self.maxhealth * .25 );
234 if (
self.health -
damage < 1 )
244 self setelectrified( 1.0 );
245 self shellshock(
"electrocution", 1.0 );
246 self playsound(
"wpn_tesla_bounce" );
251 self endon(
"disconnect" );
253 if(!IsDefined (level.one_emo_at_a_time))
255 level.one_emo_at_a_time = 0;
256 level.var_counter = 0;
258 if(level.one_emo_at_a_time == 0)
260 level.var_counter ++;
261 level.one_emo_at_a_time = 1;
262 org =
spawn(
"script_origin",
self.origin);
264 org PlaySoundWithNotify (emotion,
"sound_complete"+
"_"+level.var_counter);
265 org waittill(
"sound_complete"+
"_"+level.var_counter);
267 level.one_emo_at_a_time = 0;
273 self endon(
"disconnect" );
285 self endon(
"disconnect" );
286 self endon(
"death" );
288 self.tesla_network_death_choke = 0;
294 self.tesla_network_death_choke = 0;
309 function tesla_zombie_damage_response( willBeKilled, inflictor, attacker,
damage, flags, meansofdeath, weapon, vpoint, vdir, sHitLoc, psOffsetTime, boneIndex, surfaceType )
function tesla_killstreak_sound()
function enemy_killed_by_tesla()
function register_zombie_damage_override_callback(func)
function arc_damage(source_enemy, player, arc_num, params=level.default_lightning_chain_params)
function tesla_sound_thread()
function waittill_any_return(string1, string2, string3, string4, string5, string6, string7)
function set_zombie_var(zvar, value, is_float=false, column=1, is_team_based=false)
function play_tesla_sound(emotion)
function debug_print(str_line)
function on_player_spawned()
function register_zombie_death_animscript_callback(func)
function spawn(v_origin=(0, 0, 0), v_angles=(0, 0, 0))
function is_weapon_included(weapon)
function loop_sound(alias, interval)
function on_spawned(func, obj)
function wait_network_frame(n_count=1)
function tesla_engine_sweets()
function tesla_network_choke()
function create_lightning_chain_params(max_arcs=5, max_enemies_killed=10, radius_start=300, radius_decay=20, head_gib_chance=75, arc_travel_time=0.11, kills_for_powerup=10, min_fx_distance=128, network_death_choke=4, should_kill_enemies=true, clientside_fx=true, arc_fx_sound=undefined, no_fx=false, prevent_weapon_kill_credit=false)
function tesla_zombie_damage_response(willBeKilled, inflictor, attacker, damage, flags, meansofdeath, weapon, vpoint, vdir, sHitLoc, psOffsetTime, boneIndex, surfaceType)
function clientNotify(event)
function tesla_damage_init(hit_location, hit_origin, player)
function player_is_in_laststand()
function create_and_play_dialog(category, subcategory, force_variant)
function result(death, attacker, mod, weapon)
function cleanup_loop_sound(loop_sound)
function tesla_pvp_thread()
function tesla_zombie_death_response()
function is_tesla_damage(mod, weapon)