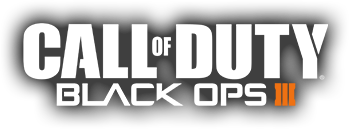 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\shared\ai\archetype_human_cover;
2 #using scripts\shared\ai\archetype_utility;
3 #using scripts\shared\ai\systems\ai_blackboard;
4 #using scripts\shared\ai\systems\animation_state_machine_notetracks;
5 #using scripts\shared\ai\systems\animation_state_machine_utility;
6 #using scripts\shared\ai\systems\behavior_tree_utility;
7 #using scripts\shared\ai\systems\blackboard;
8 #using scripts\shared\ai\systems\gib;
9 #using scripts\shared\ai\systems\shared;
10 #using scripts\shared\ai_shared;
12 #insert scripts\shared\shared.gsh;
13 #insert scripts\shared\ai\aivsaimelee.gsh;
14 #insert scripts\shared\ai\systems\animation_state_machine.gsh;
15 #insert scripts\shared\ai\systems\blackboard.gsh;
16 #insert scripts\shared\ai\systems\behavior.gsh;
17 #insert scripts\shared\ai\systems\behavior_tree.gsh;
19 #namespace AnimationStateNetwork;
57 if( entity HasPath() )
59 entity PathMode(
"move delayed",
true, RandomFloatRange( 2, 4 ) );
81 if( entity.weapon == level.weaponNone )
84 entity.lastWeapon = entity.weapon;
85 primaryweapon = entity.primaryweapon;
86 secondaryweapon = entity.secondaryweapon;
94 if( !
IS_TRUE( entity._ai_melee_attachedKnife ) )
97 entity._ai_melee_attachedKnife =
true;
103 if(
IS_TRUE( entity._ai_melee_attachedKnife ) )
106 entity._ai_melee_attachedKnife =
false;
133 if( IsActor( entity ) && entity IsInScriptedState() )
135 entity.overrideActorDamage = undefined;
136 entity.allowdeath =
true;
137 entity.skipdeath =
true;
143 entity StartRagdoll();
150 if ( IsDefined( entity ) && !entity IsRagdoll() )
152 entity StartRagdoll();
158 if( IsDefined( entity._ai_melee_opponent ) )
160 entity._ai_melee_opponent Unlink();
170 if ( IsActor( animationEntity ) && animationEntity IsInScriptedState() )
172 if( animationEntity.weapon != level.weaponNone )
174 animationEntity notify(
"about_to_shoot");
176 startPos = animationEntity GetTagOrigin(
"tag_flash" );
177 endPos = startPos + VectorScale( animationEntity GetWeaponForwardDir(), 100 );
178 MagicBullet( animationEntity.weapon, startPos, endPos, animationEntity );
180 animationEntity notify(
"shoot");
181 animationEntity.bulletsInClip--;
200 animationEntity GrenadeThrow();
202 else if ( IsDefined( animationEntity.grenadeThrowPosition ) )
206 throw_vel = animationEntity CanThrowGrenadePos( arm_offset, animationEntity.grenadeThrowPosition );
208 if ( IsDefined( throw_vel ) )
210 animationEntity GrenadeThrow();
217 if( IsDefined( animationEntity ) && IsDefined( animationEntity.enemy ) )
219 if(
IS_TRUE( animationEntity.enemy._ai_melee_markedDead ) )
221 animationEntity unlink();
function private notetrackStaircaseStep1(entity)
function private notetrackHideWeapon(entity)
function private notetrackHideAI(entity)
#define STAIRCASE_NUM_STEPS
#define NOTETRACK_RAGDOLL_NODEATH
function GibLeftArm(entity)
#define NOTETRACK_GIB_ARM_RIGHT
function private notetrackStartRagdoll(entity)
function private notetrackMeleeUnsync(animationEntity)
#define NOTETRACK_DROPGUN
#define NOTETRACK_STAIRS_STEP1
#define ASM_REGISTER_NOTETRACK_HANDLER(notetrackname, handlerfunction)
#define NOTETRACK_GIB_DISABLE
#define ASM_REGISTER_BLACKBOARD_NOTETRACK_HANDLER(notetrackName, blackboardAttributeName, blackBoardValue)
#define NOTETRACK_GIB_LEG_LEFT
function TEMP_get_arm_offset(behaviorTreeEntity, throwPosition)
function SetBlackBoardAttribute(entity, attributeName, attributeValue)
#define NOTETRACK_DROP_GUN_1
function GetBlackBoardAttribute(entity, attributeName)
#define STANCE_PRONE_ON_FRONT
#define NOTETRACK_FIRE_BULLET
function private notetrackDetachKnife(entity)
function GibRightLeg(entity)
#define NOTETRACK_GIB_ARM_LEFT
function private notetrackGrenadeThrow(animationEntity)
function GibLeftLeg(entity)
function private notetrackShowWeapon(entity)
#define NOTETRACK_GIB_HEAD
#define NOTETRACK_STANCE_STAND
function has_behavior_attribute(attribute)
function private notetrackDropGun(animationEntity)
#define NOTETRACK_HIDE_WEAPON
#define NOTETRACK_DETACH_KNIFE
#define NOTETRACK_GIB_LEG_RIGHT
function dropRiotshield(behaviorTreeEntity)
#define NOTETRACK_SHOW_WEAPON
function private notetrackAttachKnife(entity)
#define NOTETRACK_ATTACH_KNIFE
#define STANCE_PRONE_ON_BACK
#define NOTETRACK_STANCE_CROUCH
#define NOTETRACK_GRENADE_THROW
#define NOTETRACK_RAGDOLL
#define NOTETRACK_MELEE_UNSYNC
#define NOTETRACK_STAIRS_STEP2
#define NOTETRACK_HIDE_AI
#define NOTETRACK_STANCE_PRONE_BACK
function private notetrackStaircaseStep2(entity)
function private notetrackDropGunInternal(entity)
#define NOTETRACK_STANCE_PRONE_FRONT
function notetrackStartRagdollNoDeath(entity)
#define NOTETRACK_SHOW_AI
function set_behavior_attribute(attribute, value)
function private notetrackDropShield(animationEntity)
function private notetrackFireBullet(animationEntity)
function autoexec RegisterDefaultNotetrackHandlerFunctions()
function shouldThrowGrenadeAtCoverCondition(behaviorTreeEntity, throwIfPossible=false)
#define NOTETRACK_MOVEMENT_STOP
function private notetrackShowAI(entity)
function GibRightArm(entity)
function GibHead(entity)
#define NOTETRACK_DROP_SHIELD
function private notetrackGibDisable(animationEntity)
function _DelayedRagdoll(entity)
function private notetrackAnimMovementStop(entity)