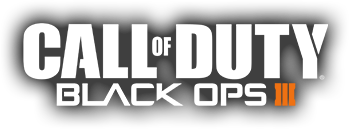 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\codescripts\struct;
3 #using scripts\shared\array_shared;
4 #using scripts\shared\audio_shared;
5 #using scripts\shared\callbacks_shared;
6 #using scripts\shared\challenges_shared;
7 #using scripts\shared\clientfield_shared;
8 #using scripts\shared\math_shared;
9 #using scripts\shared\killstreaks_shared;
10 #using scripts\shared\statemachine_shared;
11 #using scripts\shared\system_shared;
12 #using scripts\shared\turret_shared;
13 #using scripts\shared\util_shared;
14 #using scripts\shared\vehicle_ai_shared;
15 #using scripts\shared\vehicle_death_shared;
16 #using scripts\shared\vehicle_shared;
17 #using scripts\shared\vehicles\_wasp;
18 #using scripts\shared\visionset_mgr_shared;
19 #using scripts\shared\scoreevents_shared;
20 #using scripts\shared\weapons\_heatseekingmissile;
22 #using scripts\mp\_util;
23 #using scripts\mp\gametypes\_shellshock;
24 #using scripts\mp\gametypes\_spawning;
25 #using scripts\mp\killstreaks\_airsupport;
26 #using scripts\mp\killstreaks\_helicopter;
27 #using scripts\mp\killstreaks\_killstreak_bundles;
28 #using scripts\mp\killstreaks\_killstreak_detect;
29 #using scripts\mp\killstreaks\_killstreak_hacking;
30 #using scripts\mp\killstreaks\_killstreaks;
31 #using scripts\mp\killstreaks\_killstreakrules;
32 #using scripts\mp\killstreaks\_qrdrone;
33 #using scripts\mp\killstreaks\_rcbomb;
34 #using scripts\mp\killstreaks\_remote_weapons;
35 #using scripts\mp\teams\_teams;
37 #insert scripts\mp\_hacker_tool.gsh;
38 #insert scripts\mp\killstreaks\_killstreaks.gsh;
39 #insert scripts\shared\clientfields.gsh;
40 #insert scripts\shared\archetype_shared\archetype_shared.gsh;
41 #insert scripts\shared\shared.gsh;
42 #insert scripts\shared\statemachine.gsh;
43 #insert scripts\shared\version.gsh;
45 #precache( "string", "mpl_killstreak_sentinel_strt" );
46 #precache( "string", "KILLSTREAK_SENTINEL_HACKED" );
47 #precache( "string", "KILLSTREAK_SENTINEL_INBOUND" );
48 #precache( "string", "KILLSTREAK_SENTINEL_NOT_AVAILABLE" );
49 #precache( "string", "KILLSTREAK_SENTINEL_EARNED" );
50 #precache( "string", "KILLSTREAK_SENTINEL_NOT_PLACEABLE" );
51 #precache( "string", "KILLSTREAK_DESTROYED_SENTINEL" );
53 #precache( "triggerstring", "KILLSTREAK_SENTINEL_USE_REMOTE" );
56 #define SENTINEL_NAME "sentinel"
57 #define INVENTORY_SENTINEL_NAME "inventory_sentinel"
58 #define SENTINEL_SHUTOWN_NOTIFY "sentinel_shutdown"
64 killstreaks::register_dialog(
SENTINEL_NAME,
"mpl_killstreak_sentinel_strt",
"sentinelDialogBundle",
"sentinelPilotDialogBundle",
"friendlySentinel",
"enemySentinel",
"enemySentinelMultiple",
"friendlySentinelHacked",
"enemySentinelHacked",
"requestSentinel",
"threatSentinel" );
80 Target_Set(
self, ( 0, 0, 0 ) );
81 self.health =
self.healthdefault;
85 self EnableAimAssist();
89 self.fovcosinebusy = 0;
90 self.vehAirCraftCollisionEnabled =
true;
96 self.do_scripted_crash =
false;
99 self.selfDestruct =
false;
101 self.enable_target_laser =
true;
102 self.aggresive_navvolume_recover =
true;
134 self endon(
"death" );
136 self.painStartTime = GetTime();
138 if ( !
IS_TRUE(
self.inpain ) && isdefined(
self.health ) &&
self.health > 0 )
143 while ( GetTime() <
self.painStartTime + time * 1000 )
145 self SetVehVelocity(
self.velocity * stablizeParam );
146 self SetAngularVelocity(
self GetAngularVelocity() * stablizeParam );
150 if ( isdefined( restoreLookPoint ) && isdefined(
self.health ) &&
self.health > 0 )
152 restoreLookEnt =
Spawn(
"script_model", restoreLookPoint );
153 restoreLookEnt SetModel(
"tag_origin" );
155 self ClearLookAtEnt();
156 self SetLookAtEnt( restoreLookEnt );
157 self setTurretTargetEnt( restoreLookEnt );
160 self ClearLookAtEnt();
161 self ClearTurretTarget();
162 restoreLookEnt
delete();
171 function drone_pain( eAttacker, damageType, hitPoint, hitDirection, hitLocationInfo, partName, weapon )
177 ang_vel =
self GetAngularVelocity();
178 ang_vel += ( RandomFloatRange( -120, -100 ), yaw_vel, RandomFloatRange( -200, 200 ) );
179 self SetAngularVelocity( ang_vel );
185 function SentinelDamageOverride( eInflictor, eAttacker, iDamage, iDFlags, sMeansOfDeath, weapon, vPoint, vDir, sHitLoc, vDamageOrigin, psOffsetTime, damageFromUnderneath, modelIndex, partName, vSurfaceNormal )
187 if( sMeansOfDeath ==
"MOD_TRIGGER_HURT" )
192 iDamage =
killstreaks::OnDamagePerWeapon(
SENTINEL_NAME, eAttacker, iDamage, iDFlags, sMeansOfDeath, weapon,
self.maxhealth, &
destroyed_cb,
self.maxhealth*0.4, &
low_health_cb, emp_damage, undefined,
true, 1.0 );
194 if( isdefined( eAttacker ) && isdefined( eAttacker.team ) && eAttacker.team !=
self.team )
196 drone_pain( eAttacker, sMeansOfDeath, vPoint, vDir, sHitLoc, partName, weapon );
198 self.damageTaken += iDamage;
204 if( isdefined( attacker ) && isdefined( attacker.team ) && attacker.team !=
self.team )
205 self.owner.dofutz =
true;
210 if(
self.playedDamaged ==
false )
213 self.playedDamaged =
true;
221 mins = ( -5, -5, 0 );
227 testangles[0] = ( 0, 0, 0 );
228 testangles[1] = ( 0, 30, 0 );
229 testangles[2] = ( 0, -30, 0 );
230 testangles[3] = ( 0, 60, 0 );
231 testangles[4] = ( 0, -60, 0 );
232 testangles[3] = ( 0, 90, 0 );
233 testangles[4] = ( 0, -90, 0 );
239 for( i = 0; i < testangles.size; i++ )
241 startPoint = origin + ( 0, 0, heightOffset );
245 trace = physicstrace( startPoint, endPoint, mins, maxs,
self, mask );
247 if( isdefined(
trace[
"entity"] ) && IsPlayer(
trace[
"entity"] ) )
250 if(
trace[
"fraction"] > bestFrac )
252 bestFrac =
trace[
"fraction"];
253 bestOrigin =
trace[
"position"];
254 bestAngles = ( 0, angles[1], 0 ) + testangles[i];
262 if( Distance2DSquared( origin, bestOrigin ) < 20 * 20 )
267 placement = SpawnStruct();
268 placement.origin =
trace[
"position"];
269 placement.angles = bestAngles;
278 assert( IsPlayer(
self ) );
281 if( !IsNavVolumeLoaded() )
283 /# IPrintLnBold(
"Error: NavVolume Not Loaded" ); #/
284 self iPrintLnBold( &
"KILLSTREAK_SENTINEL_NOT_AVAILABLE" );
288 if( player IsPlayerSwimming() )
290 self iPrintLnBold( &
"KILLSTREAK_SENTINEL_NOT_PLACEABLE" );
295 if( !isdefined( spawnPos ) )
297 self iPrintLnBold( &
"KILLSTREAK_SENTINEL_NOT_PLACEABLE" );
307 player AddWeaponStat( GetWeapon(
"sentinel" ),
"used", 1 );
313 sentinel.killstreak_id = killstreak_id;
315 sentinel.original_vehicle_type = sentinel.vehicletype;
316 sentinel.ignore_vehicle_underneath_splash_scalar =
true;
320 sentinel.soundmod =
"player";
324 sentinel.health = sentinel.maxhealth;
327 sentinel.playedDamaged =
false;
328 sentinel.treat_owner_damage_as_friendly_fire =
true;
329 sentinel.ignore_team_kills =
true;
333 sentinel.goalHeight = 500;
335 sentinel.enable_guard =
true;
336 sentinel.always_face_enemy =
true;
360 sentinel.owner unlink();
362 if( sentinel.controlled ===
true )
374 sentinel notify(
"WatchRemoteControlDeactivate_remoteWeapons");
387 sentinel endon(
"death" );
389 level waittill(
"game_ended");
391 sentinel.abandoned =
true;
392 sentinel.selfDestruct =
true;
399 assert( IsPlayer( player ) );
401 sentinel UseVehicle( player, 0 );
407 sentinel.inHeliProximity =
false;
408 sentinel.treat_owner_damage_as_friendly_fire =
false;
409 sentinel.ignore_team_kills =
false;
411 minHeightOverride = undefined;
412 minz_struct =
struct::get(
"vehicle_oob_minz",
"targetname");
413 if( isdefined( minz_struct ) )
414 minHeightOverride = minz_struct.origin[2];
422 if ( isdefined( sentinel.PlayerDrivenVersion ) )
423 sentinel SetVehicleType( sentinel.PlayerDrivenVersion );
428 sentinel.treat_owner_damage_as_friendly_fire =
true;
429 sentinel.ignore_team_kills =
true;
431 if ( isdefined( sentinel.owner ) )
434 if( sentinel.controlled ===
true )
438 if( exitRequestedByOwner )
440 if ( isdefined( sentinel.owner ) )
443 sentinel.owner unlink();
449 if ( isdefined( sentinel.original_vehicle_type ) )
450 sentinel SetVehicleType( sentinel.original_vehicle_type );
462 if( isdefined( sentinel.owner ) )
464 RadiusDamage( sentinel.origin,
465 params.ksExplosionOuterRadius,
466 params.ksExplosionInnerDamage,
467 params.ksExplosionOuterDamage,
471 if( isdefined( params.ksExplosionRumble ) )
472 sentinel.owner PlayRumbleOnEntity( params.ksExplosionRumble );
480 self endon(
"death" );
484 if( isdefined( params.fxLowHealth ) )
488 if(
self.lowhealth >
self.health )
490 PlayFXOnTag( params.fxLowHealth,
self,
"tag_origin" );
508 sentinel waittill(
"death", attacker, damageFromUnderneath, weapon, point, dir, modType );
511 attacker =
self [[ level.figure_out_attacker ]]( attacker );
512 if ( isdefined( attacker ) && ( !isdefined(
self.owner ) ||
self.owner
util::IsEnemyPlayer( attacker ) ) )
514 if ( isPlayer( attacker ) )
518 attacker AddWeaponStat( weapon,
"destroy_aitank_or_setinel", 1 );
520 if ( modType ==
"MOD_RIFLE_BULLET" || modType ==
"MOD_PISTOL_BULLET" )
522 attacker addPlayerStat(
"shoot_down_sentinel", 1 );
524 LUINotifyEvent( &
"player_callout", 2, &
"KILLSTREAK_DESTROYED_SENTINEL", attacker.entnum );
527 if ( isdefined( sentinel ) && isdefined( sentinel.owner ) )
536 self notify(
"Sentinel_WatchTeamChange_Singleton" );
537 self endon (
"Sentinel_WatchTeamChange_Singleton" );
545 #define SENTINEL_IN_WATER_TRACE_MINS ( -2, -2, -2 )
546 #define SENTINEL_IN_WATER_TRACE_MAXS ( 2, 2, 2 )
547 #define SENTINEL_IN_WATER_TRACE_MASK ( PHYSICS_TRACE_MASK_WATER )
548 #define SENTINEL_IN_WATER_TRACE_WAIT ( 0.1 )
559 if(
trace[
"fraction"] < 1.0 )
572 if(
IS_TRUE( sentinel.control_initiated ) ||
IS_TRUE( sentinel.controlled ) )
575 while(
IS_TRUE( sentinel.control_initiated ) ||
IS_TRUE( sentinel.controlled ) )
579 if( isdefined( sentinel.owner ) )
586 if( isalive( sentinel ) )
function monitor_missiles_locked_on_to_me(player, wait_time=0.1)
function processScoreEvent(event, player, victim, weapon)
function UseRemoteWeapon(weapon, weaponName, immediate, allowManualDeactivation=true, always_allow_ride=false)
function add_interrupt_connection(from_state_name, to_state_name, on_notify, checkfunc)
function set_static(val)
function add_state(name, enter_func, update_func, exit_func, reenter_func)
#define SENTINEL_HIDE_COMPASS_ON_REMOTE_CONTROL
function WatchShutdown()
function WaitForTimeout(killstreak, duration, callback, endCondition1, endCondition2, endCondition3)
function SentinelDistanceFailure()
function state_combat_enter(params)
#define SENTINEL_SHUTOWN_NOTIFY
function drone_pain_for_time(time, stablizeParam, restoreLookPoint, weapon)
function driving_enter(params)
function StartSentinelRemoteControl(sentinel)
function ramp_in_out_thread_per_player_death_shutdown(player, ramp_in, full_period, ramp_out)
function stop_monitor_missiles_locked_on_to_me()
function nudge_collision()
function MissileTarget_ProximityDetonateIncomingMissile(endon1, endon2, allowDirectDamage)
#define SENTINEL_HOVER_RADIUS
function play_killstreak_start_dialog(killstreakType, team, killstreakId)
function state_combat_update(params)
function defaultstate_driving_enter(params)
function WatchTeamChange()
function play_pilot_dialog_on_owner(dialogKey, killstreakType, killstreakId)
function OnDamagePerWeapon(killstreak_ref, attacker, damage, flags, type, weapon, max_health, destroyed_callback, low_health, low_health_callback, emp_damage, emp_callback, allow_bullet_damage, chargeLevel)
#define SENTINEL_EMP_DAMAGE_PERCENTAGE
#define RCBOMB_PLACMENT_FROM_PLAYER
function trace(from, to, target)
function addFlySwatterStat(weapon, aircraft)
function SentinelDamageOverride(eInflictor, eAttacker, iDamage, iDFlags, sMeansOfDeath, weapon, vPoint, vDir, sHitLoc, vDamageOrigin, psOffsetTime, damageFromUnderneath, modelIndex, partName, vSurfaceNormal)
function get(kvp_value, kvp_key="targetname")
function state_guard_enter(params)
function ActivateSentinel(killstreakType)
function state_death_update(params)
function RegisterRemoteWeapon(weaponName, hintString, useCallback, endUseCallback, hideCompassOnUse=true)
function get_low_health(killstreakType)
function sndUpdateVehicleContext(added)
function IsEnemyPlayer(player)
function QRDrone_watch_distance()
function RemoveAndAssignNewRemoteControlTrigger(remoteControlTrigger)
function GetPlacementStartHeight()
function StartInitialState(defaultState="combat")
function set_vehicle_drivable_time(duration_ms, end_time_ms)
#define SENTINEL_IN_WATER_TRACE_WAIT
function drone_pain(eAttacker, damageType, hitPoint, hitDirection, hitLocationInfo, partName, weapon)
#define SENTINEL_SPAWN_Z_OFFSET
function CalcSpawnOrigin(origin, angles)
function destroyedAircraft(attacker, weapon, playerControlled)
function low_health_cb(attacker, weapon)
function friendly_fire_shield()
function play_destroyed_dialog_on_owner(killstreakType, killstreakId)
#define SENTINEL_VISIONSET_ALIAS
#define SENTINEL_VEHICLE_NAME
function init_state_machine_for_role(rolename)
function waittill_any(str_notify1, str_notify2, str_notify3, str_notify4, str_notify5)
#define SENTINEL_IN_WATER_TRACE_MINS
function get_max_health(killstreakType)
function EndSentinelRemoteControl(sentinel, exitRequestedByOwner)
#define SENTINEL_IN_WATER_TRACE_MASK
function ConfigureTeamPost(owner, isHacked)
function register_dialog(killstreakType, informDialog, taacomDialogBundleKey, pilotDialogArrayKey, startDialogKey, enemyStartDialogKey, enemyStartMultipleDialogKey, hackedDialogKey, hackedStartDialogKey, requestDialogKey, threatDialogKey, isInventory)
function state_guard_exit(params)
#define INVALID_KILLSTREAK_ID
#define SENTINEL_DURATION
#define PHYSICS_TRACE_MASK_PHYSICS
#define PHYSICS_TRACE_MASK_VEHICLE
function set_vehicle_drivable_time_starting_now(killstreak, duration_ms=(-1))
function add_to_target_group(target_ent)
function Spawn(parent, onDeathCallback)
#define SENTINEL_MAX_DISTANCE_FROM_OWNER
function destroyed_cb(attacker, weapon)
#define SENTINEL_MISSILES_TO_DESTROY
function register_strings(killstreakType, receivedText, notUsableText, inboundText, inboundNearPlayerText, hackedText, utilizesAirspace=true, isInventory=false)
function killstreakStop(hardpointType, team, id)
function state_guard_update(params)
function get_hacked_health(killstreakType)
function init_guard_points()
function register_info(type, name, version, lerp_step_count)
#define SENTINEL_HOVER_SPEED
#define SENTINEL_IN_WATER_TRACE_MAXS
#define SENTINEL_MAX_HEIGHT_OFFSET
function killstreakStart(hardpointType, team, hacked, displayTeamMessage)
function state_guard_can_enter(from_state, to_state, connection)
function set(str_field_name, n_value)
function HackedCallbackPost(hacker)
#define SENTINEL_HOVER_ACCELERATION
function vehicleSpawnContext()
function configure_team(killstreakType, killstreakId, owner, influencerType, configureTeamPreFunction, configureTeamPostFunction, isHacked=false)
function add_utility_connection(from_state_name, to_state_name, checkfunc, defaultScore)
function EndRemoteControlWeaponUse(exitRequestedByOwner)
function register_alt_weapon(killstreakType, weaponName, isInventory)
function create_flare_ent(offset)
function WatchGameEnded()
function get_script_bundle(str_type, str_name)
function init_target_group()
function enable_hacking(killstreakName, preHackFunction, postHackFunction)
function HackedCallbackPre(hacker)
#define SENTINEL_NEAR_GOAL_NOTIFY_DIST
function HealthMonitor()
function get_state_callbacks(statename)
#define WAIT_SERVER_FRAME