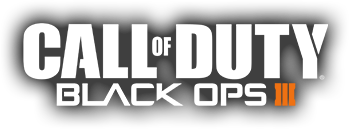 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\shared\system_shared;
2 #using scripts\shared\clientfield_shared;
3 #using scripts\shared\callbacks_shared;
4 #using scripts\shared\util_shared;
5 #using scripts\shared\flag_shared;
6 #using scripts\shared\vehicle_shared;
7 #using scripts\shared\music_shared;
9 #insert scripts\shared\shared.gsh;
10 #insert scripts\shared\version.gsh;
44 level waittill(
"chyron_menu_open" );
47 level waittill(
"chyron_menu_closed" );
54 level waittill(
"scene_skip_sequence_started" );
55 music::setmusicstate(
"death" );
61 self util::clientnotify(
"sndDEDe" );
67 self util::clientnotify(
"sndDED" );
76 if( !isdefined(
self.sndOccupants ) )
78 self.sndOccupants = 0;
88 if(
self.sndOccupants < 0 )
90 self.sndOccupants = 0;
99 self notify(
"stop_target_missile_sound");
100 self endon(
"stop_target_missile_sound" );
101 self endon(
"disconnect" );
102 self endon(
"death" );
104 if (IsDefined(alias))
106 time = SoundGetPlaybackTime(alias)*0.001;
111 self playLocalSound( alias );
122 self endon(
"disconnect");
126 self flag::init(
"playing_stinger_fired_at_me",
false);
135 self waittill(
"missile_lock", attacker, weapon);
136 if (!
flag::get(
"playing_stinger_fired_at_me"))
140 self notify(
"stop_target_missile_sound");
148 self endon(
"disconnect");
153 self waittill(
"stinger_fired_at_me",missile, weapon ,attacker);
155 self flag::set(
"playing_stinger_fired_at_me");
158 self notify(
"stop_target_missile_sound");
168 if( isdefined(level.players) && level.players.size > 0 )
170 foreach( player
in level.players )
172 player UnlockSongByAlias(unlockName);
178 self UnlockSongByAlias(unlockName);
function register_clientfields()
function set_to_player(str_field_name, n_value)
function clear(str_flag)
function missileLockWatcher()
function sndResetSoundSettings()
function get(kvp_value, kvp_key="targetname")
function sndUpdateVehicleContext(added)
function PlayTargetMissileSound(alias, looping)
function sndChyronWatcher()
function on_spawned(func, obj)
function missileFireWatcher()
function waittill_any(str_notify1, str_notify2, str_notify3, str_notify4, str_notify5)
function killcam(attackerNum, targetNum, killcam_entity_info, weapon, meansOfDeath, deathTime, deathTimeOffset, offsetTime, respawn, maxtime, perks, killstreaks, attacker, keep_deathcam)
#define REGISTER_SYSTEM(__sys, __func_init_preload, __reqs)
function unlockFrontendMusic(unlockName, allplayers=true)
function on_vehicle_spawned()
function set(str_field_name, n_value)
function exists(str_flag)
function vehicleSpawnContext()
function on_player_killed()
function sndIGCskipWatcher()