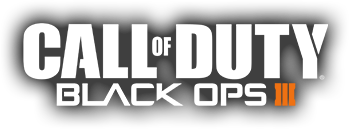 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\codescripts\struct;
2 #using scripts\shared\callbacks_shared;
3 #using scripts\shared\clientfield_shared;
4 #using scripts\shared\flagsys_shared;
5 #using scripts\shared\abilities\_ability_gadgets;
6 #using scripts\shared\abilities\_ability_player;
7 #using scripts\shared\abilities\_ability_util;
9 #insert scripts\shared\shared.gsh;
10 #insert scripts\shared\version.gsh;
11 #insert scripts\shared\abilities\_ability_util.gsh;
13 #using scripts\shared\system_shared;
41 return self GadgetFlickering( slot );
46 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
48 self [[level.cybercom.active_camo._on_connect]]();
54 if ( IsDefined(
self.sound_ent ) )
56 self.sound_ent stoploopsound( .05 );
57 self.sound_ent
delete();
64 self notify(
"camo_off" );
69 if ( IsDefined(
self.sound_ent ) )
71 self.sound_ent stoploopsound( .05 );
72 self.sound_ent
delete();
85 self endon(
"disconnect" );
86 self endon(
"camo_off" );
100 self endon(
"death" );
101 self endon(
"camo_off" );
111 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
113 self [[level.cybercom.active_camo._on_give]](slot, weapon);
119 self notify(
"camo_removed" );
120 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
122 self [[level.cybercom.active_camo._on_take]](slot, weapon);
129 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
131 self thread [[level.cybercom.active_camo._on_flicker]](slot, weapon);
137 str_opposite_team =
"axis";
139 if (
self.team ==
"axis" )
141 str_opposite_team =
"allies";
144 aiTargets = GetAIArray( str_opposite_team );
146 for ( i = 0; i < aiTargets.size; i++ )
148 testTarget = aiTargets[i];
150 if ( !IsDefined( testTarget ) || !IsAlive( testTarget ) )
162 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
164 self thread [[level.cybercom.active_camo._on]](slot, weapon);
169 self._gadget_camo_oldIgnoreme =
self.ignoreme;
170 self.ignoreme =
true;
202 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
204 self thread [[level.cybercom.active_camo._off]](slot, weapon);
206 if ( IsDefined(
self.sound_ent ) )
216 self notify(
"camo_off" );
218 if ( IsDefined(
self._gadget_camo_oldIgnoreme ) )
220 self.ignoreme =
self._gadget_camo_oldIgnoreme;
221 self._gadget_camo_oldIgnoreme = undefined;
225 self.ignoreme =
false;
230 self.gadget_camo_off_time = GetTime();
238 self notify(
"camo_bread_crumb" );
239 self endon(
"camo_bread_crumb" );
248 self._camo_crumb =
spawn(
"script_model",
self.origin );
249 self._camo_crumb SetModel(
"tag_origin" );
261 self endon(
"disconnect" );
262 self endon(
"death" );
263 self endon(
"camo_off" );
264 self endon(
"camo_bread_crumb" );
270 currentTime = GetTime();
272 if ( currentTime -
startTime >
self._gadgets_player[slot].gadget_breadCrumbDuration )
283 if ( IsDefined(
self._camo_crumb ) )
285 self._camo_crumb
delete();
286 self._camo_crumb = undefined;
292 self endon(
"disconnect" );
293 self endon(
"camo_off" );
297 self waittill(
"weapon_assassination" );
301 if (
self._gadgets_player[slot].gadget_takedownrevealtime > 0 )
311 self endon(
"disconnect" );
318 self notify(
"temporary_dont_ignore" );
322 old_ignoreme =
false;
324 if ( IsDefined(
self._gadget_camo_oldIgnoreme ) )
326 old_ignoreme =
self._gadget_camo_oldIgnoreme;
329 self.ignoreme = old_ignoreme;
338 self endon(
"disconnect" );
339 self endon(
"death" );
340 self endon(
"camo_off" );
341 self endon(
"temporary_dont_ignore" );
356 self endon(
"disconnect" );
357 self endon(
"death" );
358 self endon(
"camo_off" );
384 self._gadget_camo_reveal_status = undefined;
386 if ( IsDefined( time ) )
388 timeStr =
", ^3time: " + time;
389 self._gadget_camo_reveal_status = status;
392 if ( GetDvarInt(
"scr_cpower_debug_prints" ) > 0 )
393 self IPrintlnBold(
"Camo Reveal: " + status + timeStr );
function register_gadget_is_flickering_callbacks(type, flickering_func)
class AnimationAdjustmentInfoZ startTime
function camo_on_spawn()
function camo_takedown_watch(slot, weapon)
function camo_on_flicker(slot, weapon)
#define GADGET_CAMO_SHADER_OFF
function register_gadget_possession_callbacks(type, on_give, on_take)
function camo_on_disconnect()
function camo_suit_flicker(slot, weapon)
#define GADGET_CAMO_SHADER_ON
function clear(str_flag)
function camo_on_connect()
function register_gadget_is_inuse_callbacks(type, inuse_func)
#define GADGET_CAMO_SHADER_FLICKER
function SetFlickering(slot, length)
function camo_gadget_on(slot, weapon)
function camo_all_actors(value)
function spawn(v_origin=(0, 0, 0), v_angles=(0, 0, 0))
function on_disconnect()
function get(kvp_value, kvp_key="targetname")
function register_gadget_flicker_callbacks(type, on_flicker)
function camo_gadget_off(slot, weapon)
function camo_bread_crumb_delete()
function camo_temporary_dont_ignore_wait(slot)
function camo_bread_crumb(slot, weapon)
function suspend_camo_suit_wait(slot, weapon)
function camo_is_flickering(slot)
function camo_temporary_dont_ignore(slot)
function on_spawned(func, obj)
function camo_is_inuse(slot)
function register_gadget_activation_callbacks(type, turn_on, turn_off)
function suspend_camo_suit(slot, weapon)
function camo_bread_crumb_wait(slot, weapon)
#define REGISTER_SYSTEM(__sys, __func_init_preload, __reqs)
function set_camo_reveal_status(status, time)
function set(str_field_name, n_value)
function camo_on_take(slot, weapon)
function camo_on_give(slot, weapon)
#define GADGET_TYPE_OPTIC_CAMO