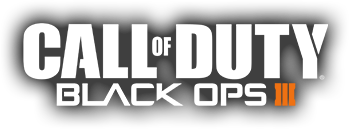 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\codescripts\struct;
2 #using scripts\shared\audio_shared;
3 #using scripts\shared\callbacks_shared;
4 #using scripts\shared\clientfield_shared;
5 #using scripts\shared\exploder_shared;
6 #using scripts\shared\scene_shared;
7 #using scripts\shared\util_shared;
9 #insert scripts\shared\shared.gsh;
10 #insert scripts\shared\version.gsh;
12 #using scripts\zm\_load;
13 #using scripts\zm\_zm_weapons;
16 #using scripts\zm\_zm_pack_a_punch;
17 #using scripts\zm\_zm_perk_additionalprimaryweapon;
18 #using scripts\zm\_zm_perk_doubletap2;
19 #using scripts\zm\_zm_perk_deadshot;
20 #using scripts\zm\_zm_perk_juggernaut;
21 #using scripts\zm\_zm_perk_quick_revive;
22 #using scripts\zm\_zm_perk_sleight_of_hand;
23 #using scripts\zm\_zm_perk_staminup;
26 #using scripts\zm\_zm_powerup_double_points;
27 #using scripts\zm\_zm_powerup_carpenter;
28 #using scripts\zm\_zm_powerup_fire_sale;
29 #using scripts\zm\_zm_powerup_free_perk;
30 #using scripts\zm\_zm_powerup_full_ammo;
31 #using scripts\zm\_zm_powerup_insta_kill;
32 #using scripts\zm\_zm_powerup_nuke;
33 #using scripts\zm\_zm_powerup_weapon_minigun;
36 #using scripts\zm\_zm_trap_electric;
39 #using scripts\zm\_zm_weap_bouncingbetty;
40 #using scripts\zm\_zm_weap_cymbal_monkey;
41 #using scripts\zm\_zm_weap_tesla;
42 #using scripts\zm\_zm_weap_rocketshield;
43 #using scripts\zm\_zm_weap_gravityspikes;
44 #using scripts\zm\_zm_weap_thundergun;
45 #using scripts\zm\_zm_weap_octobomb;
46 #using scripts\zm\_zm_weap_raygun_mark3;
49 #using scripts\zm\_zm_ai_dogs;
51 #namespace zm_usermap;
53 #precache( "client_fx", "zombie/fx_glow_eye_orange" );
54 #precache( "client_fx", "zombie/fx_bul_flesh_head_fatal_zmb" );
55 #precache( "client_fx", "zombie/fx_bul_flesh_head_nochunks_zmb" );
56 #precache( "client_fx", "zombie/fx_bul_flesh_neck_spurt_zmb" );
57 #precache( "client_fx", "zombie/fx_blood_torso_explo_zmb" );
58 #precache( "client_fx", "trail/fx_trail_blood_streak" );
59 #precache( "client_fx", "dlc0/factory/fx_snow_player_os_factory" );
72 level._effect[
"eye_glow"] =
"zombie/fx_glow_eye_orange";
73 level._effect[
"headshot"] =
"zombie/fx_bul_flesh_head_fatal_zmb";
74 level._effect[
"headshot_nochunks"] =
"zombie/fx_bul_flesh_head_nochunks_zmb";
75 level._effect[
"bloodspurt"] =
"zombie/fx_bul_flesh_neck_spurt_zmb";
77 level._effect[
"animscript_gib_fx"] =
"zombie/fx_blood_torso_explo_zmb";
78 level._effect[
"animscript_gibtrail_fx"] =
"trail/fx_trail_blood_streak";
81 level.debug_keyline_zombies =
false;
98 #define JUGGERNAUT_MACHINE_LIGHT_FX "jugger_light"
99 #define QUICK_REVIVE_MACHINE_LIGHT_FX "revive_light"
100 #define STAMINUP_MACHINE_LIGHT_FX "marathon_light"
101 #define WIDOWS_WINE_FX_MACHINE_LIGHT "widow_light"
102 #define SLEIGHT_OF_HAND_MACHINE_LIGHT_FX "sleight_light"
103 #define DOUBLETAP2_MACHINE_LIGHT_FX "doubletap2_light"
104 #define DEADSHOT_MACHINE_LIGHT_FX "deadshot_light"
105 #define ADDITIONAL_PRIMARY_WEAPON_MACHINE_LIGHT_FX "additionalprimaryweapon_light"
121 level.exert_sounds[1][
"falldamage"][0] =
"vox_plr_0_exert_pain_0";
122 level.exert_sounds[1][
"falldamage"][1] =
"vox_plr_0_exert_pain_1";
123 level.exert_sounds[1][
"falldamage"][2] =
"vox_plr_0_exert_pain_2";
124 level.exert_sounds[1][
"falldamage"][3] =
"vox_plr_0_exert_pain_3";
125 level.exert_sounds[1][
"falldamage"][4] =
"vox_plr_0_exert_pain_4";
127 level.exert_sounds[2][
"falldamage"][0] =
"vox_plr_1_exert_pain_0";
128 level.exert_sounds[2][
"falldamage"][1] =
"vox_plr_1_exert_pain_1";
129 level.exert_sounds[2][
"falldamage"][2] =
"vox_plr_1_exert_pain_2";
130 level.exert_sounds[2][
"falldamage"][3] =
"vox_plr_1_exert_pain_3";
131 level.exert_sounds[2][
"falldamage"][4] =
"vox_plr_1_exert_pain_4";
133 level.exert_sounds[3][
"falldamage"][0] =
"vox_plr_2_exert_pain_0";
134 level.exert_sounds[3][
"falldamage"][1] =
"vox_plr_2_exert_pain_1";
135 level.exert_sounds[3][
"falldamage"][2] =
"vox_plr_2_exert_pain_2";
136 level.exert_sounds[3][
"falldamage"][3] =
"vox_plr_2_exert_pain_3";
137 level.exert_sounds[3][
"falldamage"][4] =
"vox_plr_2_exert_pain_4";
139 level.exert_sounds[4][
"falldamage"][0] =
"vox_plr_3_exert_pain_0";
140 level.exert_sounds[4][
"falldamage"][1] =
"vox_plr_3_exert_pain_1";
141 level.exert_sounds[4][
"falldamage"][2] =
"vox_plr_3_exert_pain_2";
142 level.exert_sounds[4][
"falldamage"][3] =
"vox_plr_3_exert_pain_3";
143 level.exert_sounds[4][
"falldamage"][4] =
"vox_plr_3_exert_pain_4";
146 level.exert_sounds[1][
"meleeswipesoundplayer"][0] =
"vox_plr_0_exert_melee_0";
147 level.exert_sounds[1][
"meleeswipesoundplayer"][1] =
"vox_plr_0_exert_melee_1";
148 level.exert_sounds[1][
"meleeswipesoundplayer"][2] =
"vox_plr_0_exert_melee_2";
149 level.exert_sounds[1][
"meleeswipesoundplayer"][3] =
"vox_plr_0_exert_melee_3";
150 level.exert_sounds[1][
"meleeswipesoundplayer"][4] =
"vox_plr_0_exert_melee_4";
152 level.exert_sounds[2][
"meleeswipesoundplayer"][0] =
"vox_plr_1_exert_melee_0";
153 level.exert_sounds[2][
"meleeswipesoundplayer"][1] =
"vox_plr_1_exert_melee_1";
154 level.exert_sounds[2][
"meleeswipesoundplayer"][2] =
"vox_plr_1_exert_melee_2";
155 level.exert_sounds[2][
"meleeswipesoundplayer"][3] =
"vox_plr_1_exert_melee_3";
156 level.exert_sounds[2][
"meleeswipesoundplayer"][4] =
"vox_plr_1_exert_melee_4";
158 level.exert_sounds[3][
"meleeswipesoundplayer"][0] =
"vox_plr_2_exert_melee_0";
159 level.exert_sounds[3][
"meleeswipesoundplayer"][1] =
"vox_plr_2_exert_melee_1";
160 level.exert_sounds[3][
"meleeswipesoundplayer"][2] =
"vox_plr_2_exert_melee_2";
161 level.exert_sounds[3][
"meleeswipesoundplayer"][3] =
"vox_plr_2_exert_melee_3";
162 level.exert_sounds[3][
"meleeswipesoundplayer"][4] =
"vox_plr_2_exert_melee_4";
164 level.exert_sounds[4][
"meleeswipesoundplayer"][0] =
"vox_plr_3_exert_melee_0";
165 level.exert_sounds[4][
"meleeswipesoundplayer"][1] =
"vox_plr_3_exert_melee_1";
166 level.exert_sounds[4][
"meleeswipesoundplayer"][2] =
"vox_plr_3_exert_melee_2";
167 level.exert_sounds[4][
"meleeswipesoundplayer"][3] =
"vox_plr_3_exert_melee_3";
168 level.exert_sounds[4][
"meleeswipesoundplayer"][4] =
"vox_plr_3_exert_melee_4";
#define SLEIGHT_OF_HAND_MACHINE_LIGHT_FX
#define QUICK_REVIVE_MACHINE_LIGHT_FX
function include_weapons()
function autoexec opt_in()
#define ADDITIONAL_PRIMARY_WEAPON_MACHINE_LIGHT_FX
#define JUGGERNAUT_MACHINE_LIGHT_FX
#define DEFAULT(__var, __default)
function load_weapon_spec_from_table(table, first_row)
function setup_personality_character_exerts()
#define DEADSHOT_MACHINE_LIGHT_FX
#define DOUBLETAP2_MACHINE_LIGHT_FX
function include_perks()
#define STAMINUP_MACHINE_LIGHT_FX