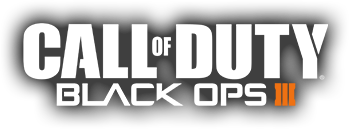 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\shared\array_shared;
3 #using scripts\shared\ai_shared;
4 #using scripts\shared\ai\systems\ai_interface;
5 #using scripts\shared\ai\systems\blackboard;
6 #using scripts\shared\ai\systems\shared;
7 #using scripts\shared\ai\archetype_utility;
8 #using scripts\shared\ai\archetype_cover_utility;
9 #using scripts\shared\ai\archetype_locomotion_utility;
11 #insert scripts\shared\ai\systems\blackboard.gsh;
12 #insert scripts\shared\ai\utility.gsh;
13 #insert scripts\shared\shared.gsh;
15 #namespace Blackboard;
49 return AngleClamp180(
self.angles[ 1 ] -
self.node.angles[ 1 ] );
52 #define CLOSE_FRIENDLY_DISTANCE ( 120 )
53 #define MAX_NEARBY_FRIENDLIES 3
64 if ( !isDefined(
self.nearbyFriendlyCheck ) )
65 self.nearbyFriendlyCheck = 0;
69 if ( now >=
self.nearbyFriendlyCheck )
72 self.nearbyFriendlyCheck = now + 500;
82 if ( IsDefined(
self.enemy ) && IsDefined(
self.runAndGunDist ) )
84 if ( DistanceSquared(
self.origin,
self LastKnownPos(
self.enemy ) )
85 > (
self.runAndGunDist *
self.runAndGunDist ) )
90 else if ( IsDefined(
self.goalpos ) && IsDefined(
self.runAndGunDist ) )
92 if ( DistanceSquared(
self.origin,
self.goalpos )
93 > (
self.runAndGunDist *
self.runAndGunDist ) )
105 if(
self ASMIsTransitionRunning() )
110 if( !IsDefined(
self.node ) )
117 if( IsDefined( coverMode ) )
119 coverNode =
self.node;
#define HUMAN_COVER_FLANKABILITY
function private BB_GetCoverFlankability()
#define HUMAN_LOCOMOTION_MOVEMENT_DEFAULT
#define COVER_BLIND_MODE
#define NODE_COVER_STAND(_node)
function private BB_GetTacticalArrivalFacingYaw()
#define HUMAN_LOCOMOTION_MOVEMENT_SPRINT
#define NODE_COVER_PILLAR(_node)
#define NODE_COVER_LEFT(_node)
function RegisterActorBlackBoardAttributes()
#define BB_REGISTER_ATTRIBUTE(name, defaultValue, getter)
function private BB_GetLocomotionMovementType()
#define CLOSE_FRIENDLY_DISTANCE
#define HUMAN_COVER_UNFLANKABLE
function GetBlackBoardAttribute(entity, attributeName)
#define HUMAN_LOCOMOTION_VARIATION
#define NODE_COVER_RIGHT(_node)
#define DONT_ARRIVE_AT_GOAL
#define MAX_NEARBY_FRIENDLIES
#define TACTICAL_ARRIVAL_FACING_YAW
function private BB_GetArrivalType()
function GetAiAttribute(entity, attribute)
#define HUMAN_LOCOMOTION_MOVEMENT_TYPE
#define COVER_ALERT_MODE
#define HUMAN_COVER_FLANKABLE
function get_behavior_attribute(attribute)
#define NODE_COVER_CROUCH(_node)