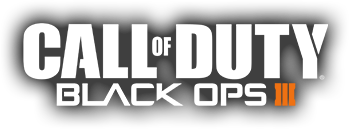 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\codescripts\struct;
3 #using scripts\shared\clientfield_shared;
4 #using scripts\shared\math_shared;
5 #using scripts\shared\system_shared;
6 #using scripts\shared\util_shared;
7 #using scripts\shared\vehicle_shared;
8 #using scripts\shared\vehicles\_driving_fx;
10 #insert scripts\mp\killstreaks\_killstreaks.gsh;
11 #insert scripts\shared\shared.gsh;
12 #insert scripts\shared\version.gsh;
14 #using scripts\mp\_callbacks;
15 #using scripts\mp\_util;
16 #using scripts\mp\_vehicle;
21 #precache( "client_fx", "killstreaks/fx_rcxd_lights_blinky" );
22 #precache( "client_fx", "killstreaks/fx_rcxd_lights_solid" );
23 #precache( "client_fx", "killstreaks/fx_rcxd_lights_red" );
24 #precache( "client_fx", "killstreaks/fx_rcxd_lights_grn" );
25 #precache( "client_fx", "_t6/weapon/grenade/fx_spark_disabled_rc_car" );
29 #define RCBOMB_BLUR_AMOUNT GetDvarFloat( "scr_rcbomb_amount", 0.1 )
30 #define RCBOMB_BLUR_INNER_RADIUS GetDvarFloat( "scr_rcbomb_inner_radius", 0.5 )
31 #define RCBOMB_BLUR_OUTER_RADIUS GetDvarFloat( "scr_rcbomb_outer_radius", 0.75 )
32 #define RCBOMB_BLUR_BOOST_DURATION GetDvarFloat( "scr_rcbomb_duration", 1.0 )
38 level._effect[
"rcbomb_enemy_light"] =
"killstreaks/fx_rcxd_lights_blinky";
39 level._effect[
"rcbomb_friendly_light"] =
"killstreaks/fx_rcxd_lights_solid";
40 level._effect[
"rcbomb_enemy_light_blink"] =
"killstreaks/fx_rcxd_lights_red";
41 level._effect[
"rcbomb_friendly_light_blink"] =
"killstreaks/fx_rcxd_lights_grn";
42 level._effect[
"rcbomb_stunned"] =
"_t6/weapon/grenade/fx_spark_disabled_rc_car";
62 self.killstreakBundle = level.rcBombBundle;
67 self endon(
"entityshutdown" );
69 if ( !IsDemoPlaying() )
83 self endon(
"entityshutdown" );
85 if ( isdefined(
self.owner ) &&
self.owner isLocalPlayer() )
91 DisableSpeedBlur( localClientNum );
97 self endon(
"entityshutdown" );
101 self waittill(
"veh_boost" );
108 self waittill(
"entityshutdown" );
109 DisableSpeedBlur( localClientNum );
166 speed =
self getspeed();
167 maxspeed =
self getmaxspeed();
171 maxspeed =
self getmaxreversespeed();
176 speed_fraction = Abs(speed) / maxspeed;
182 if (
self iswheelcolliding(
"back_left" ) ||
self iswheelcolliding(
"back_right" ) )
185 if (
self IsLocalClientDriver( localClientNum ) )
194 self endon(
"entityshutdown" );
198 speed =
self GetSpeed();
202 self PlaySound( localClientNum,
"mpl_veh_rc_boost" );
212 self endon(
"entityshutdown" );
218 self waittill(
"stunned" );
223 self setstunned(
true );
233 self endon(
"entityshutdown" );
234 self endon(
"stunned" );
236 self waittill(
"not_stunned" );
241 self setstunned(
false );
246 self endon(
"entityshutdown" );
247 self endon(
"stunned" );
248 self endon(
"not_stunned" );
253 playfxontag( localClientNum, level._effect[
"rcbomb_stunned"],
self,
"tag_origin" );
260 self endon(
"entityshutdown" );
264 self waittill(
"veh_engine_stutter" );
265 if (
self IsLocalClientDriver( localClientNum ) )
267 player = getlocalplayer( localClientNum );
269 if( isdefined( player ) )
271 player PlayRumbleOnEntity( localClientNum,
"rcbomb_engine_stutter" );
279 if( isdefined( hit_intensity ) && hit_intensity > 15 )
283 if (isdefined (
self.sounddef))
285 alias =
self.sounddef +
"_suspension_lg_hd";
289 alias =
"veh_default_suspension_lg_hd";
292 id = PlaySound( 0, alias,
self.origin, volume);
293 player Earthquake( 0.7, 0.25, player.origin, 1500 );
294 player PlayRumbleOnEntity( localClientNum,
"damage_heavy" );
#define RCBOMB_BLUR_OUTER_RADIUS
function play_driving_screen_fx(localClientNum)
function lights_off(localClientNum)
#define RCBOMB_BLUR_BOOST_DURATION
function boost_think(localClientNum)
#define RCBOMB_BLUR_AMOUNT
#define CF_CALLBACK_ZERO_ON_NEW_ENT
function engineStutterHandler(localClientNum)
function play_stunned_fx_handler(localClientNum)
function boost_blur(localClientNum)
function play_boost_fx(localClientNum)
#define RCBOMB_BLUR_INNER_RADIUS
function waittill_any(str_notify1, str_notify2, str_notify3, str_notify4, str_notify5)
function OnDrivingFxJumpLanding(localClientNum, player)
#define REGISTER_SYSTEM(__sys, __func_init_preload, __reqs)
function demo_think(localClientNum)
function stunnedHandler(localClientNum)
function play_screen_fx_dust(localClientNum)
function add_vehicletype_callback(vehicletype, callback)
function spawned(localClientNum)
function get_impact_vol_from_speed()
function get_script_bundle(str_type, str_name)
function notStunnedHandler(localClientNum)
function server_wait(localClientNum, seconds, waitBetweenChecks, level_endon)
function shutdown_think(localClientNum)
function play_screen_fx_dirt(localClientNum)
function callback_stunned(localClientNum, oldVal, newVal, bNewEnt, bInitialSnap, fieldName, bWasTimeJump)
function OnDrivingFxCollision(localClientNum, player, hip, hitn, hit_intensity)