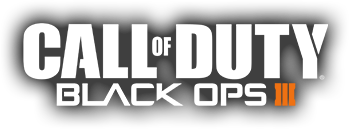 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\codescripts\struct;
2 #using scripts\shared\callbacks_shared;
3 #using scripts\shared\clientfield_shared;
4 #using scripts\shared\flagsys_shared;
5 #using scripts\shared\abilities\_ability_gadgets;
6 #using scripts\shared\abilities\_ability_player;
7 #using scripts\shared\abilities\_ability_util;
9 #insert scripts\shared\shared.gsh;
10 #insert scripts\shared\version.gsh;
11 #insert scripts\shared\abilities\_ability_util.gsh;
13 #using scripts\shared\system_shared;
32 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
34 self [[level.cybercom.active_camo._on_connect]]();
48 self notify(
"camo_off" );
66 return self GadgetFlickering( slot );
71 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
73 self [[level.cybercom.active_camo._on_give]](slot, weapon);
79 self notify(
"camo_removed" );
80 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
82 self [[level.cybercom.active_camo._on_take]](slot, weapon);
89 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
91 self thread [[level.cybercom.active_camo._on_flicker]](slot, weapon);
97 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
99 self thread [[level.cybercom.active_camo._on]](slot, weapon);
112 if(isDefined(level.cybercom) && isDefined(level.cybercom.active_camo))
114 self thread [[level.cybercom.active_camo._off]](slot, weapon);
121 self notify(
"camo_off" );
128 self endon(
"disconnect" );
129 self endon(
"camo_off" );
143 self endon(
"death" );
144 self endon(
"camo_off" );
function register_gadget_is_flickering_callbacks(type, flickering_func)
function camo_is_flickering(slot)
#define GADGET_CAMO_SHADER_OFF
#define GADGET_TYPE_ACTIVE_CAMO
function register_gadget_possession_callbacks(type, on_give, on_take)
#define GADGET_CAMO_SHADER_ON
function clear(str_flag)
function register_gadget_is_inuse_callbacks(type, inuse_func)
#define GADGET_CAMO_SHADER_FLICKER
function camo_on_spawn()
function on_disconnect()
function get(kvp_value, kvp_key="targetname")
function camo_is_inuse(slot)
function register_gadget_flicker_callbacks(type, on_flicker)
function suspend_camo_suit(slot, weapon)
function camo_on_flicker(slot, weapon)
function on_spawned(func, obj)
function suspend_camo_suit_wait(slot, weapon)
function register_gadget_activation_callbacks(type, turn_on, turn_off)
function camo_gadget_on(slot, weapon)
function camo_on_disconnect()
#define REGISTER_SYSTEM(__sys, __func_init_preload, __reqs)
function camo_on_connect()
function camo_gadget_off(slot, weapon)
function set(str_field_name, n_value)
function camo_on_take(slot, weapon)
function camo_on_give(slot, weapon)