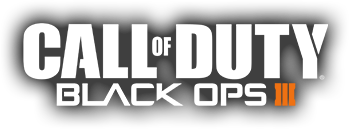 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\codescripts\struct;
3 #using scripts\shared\challenges_shared;
4 #using scripts\shared\clientfield_shared;
5 #using scripts\shared\scoreevents_shared;
6 #using scripts\shared\util_shared;
8 #using scripts\mp\_challenges;
9 #using scripts\mp\_util;
10 #using scripts\mp\gametypes\_spawning;
11 #using scripts\mp\killstreaks\_airsupport;
12 #using scripts\mp\killstreaks\_killstreakrules;
13 #using scripts\mp\killstreaks\_killstreaks;
14 #using scripts\mp\killstreaks\_satellite;
15 #using scripts\mp\killstreaks\_planemortar;
16 #using scripts\mp\killstreaks\_killstreak_bundles;
17 #using scripts\mp\gametypes\_globallogic_audio;
18 #using scripts\shared\weapons\_hacker_tool;
19 #using scripts\mp\killstreaks\_killstreak_hacking;
21 #insert scripts\mp\_hacker_tool.gsh;
22 #insert scripts\mp\killstreaks\_killstreaks.gsh;
23 #insert scripts\shared\shared.gsh;
24 #insert scripts\shared\version.gsh;
26 #namespace drone_strike;
27 #define DRONE_STRIKE_NAME "drone_strike"
29 #precache( "locationselector", "map_directional_selector" );
30 #precache( "string", "KILLSTREAK_DRONE_STRIKE_NOT_AVAILABLE" );
31 #precache( "string", "KILLSTREAK_DRONE_STRIKE_EARNED" );
32 #precache( "string", "KILLSTREAK_DRONE_STRIKE_INBOUND" );
33 #precache( "string", "KILLSTREAK_DRONE_STRIKE_INBOUND_NEAR_PLAYER" );
34 #precache( "string", "KILLSTREAK_DRONE_STRIKE_HACKED" );
35 #precache( "string", "KILLSTREAK_DESTROYED_ROLLING_THUNDER_DRONE" );
36 #precache( "string", "KILLSTREAK_DESTROYED_ROLLING_THUNDER_ALL_DRONES" );
38 #precache( "eventstring", "mpl_killstreak_DRONE_STRIKE" );
39 #precache( "fx", "killstreaks/fx_rolling_thunder_thruster_trails" );
44 killstreaks::register_strings(
DRONE_STRIKE_NAME, &
"KILLSTREAK_DRONE_STRIKE_EARNED", &
"KILLSTREAK_DRONE_STRIKE_NOT_AVAILABLE", &
"KILLSTREAK_DRONE_STRIKE_INBOUND", &
"KILLSTREAK_DRONE_STRIKE_INBOUND_NEAR_PLAYER", &
"KILLSTREAK_DRONE_STRIKE_HACKED" );
45 killstreaks::register_dialog(
DRONE_STRIKE_NAME,
"mpl_killstreak_drone_strike",
"droneStrikeDialogBundle", undefined,
"friendlyDroneStrike",
"enemyDroneStrike",
"enemyDroneStrikeMultiple",
"friendlyDroneStrikeHacked",
"enemyDroneStrikeHacked",
"requestDroneStrike",
"threatDroneStrike" );
69 self.selectingLocation =
true;
70 self thread airsupport::EndSelectionThink();
73 if( !isdefined(
self.pers[
"drone_strike_radar_used"] ) || !
self.pers[
"drone_strike_radar_used"] )
75 self thread planemortar::SingleRadarSweep();
81 if( !isdefined(
self ) )
86 if ( !isdefined( location.origin ) )
88 self.pers[
"drone_strike_radar_used"] =
true;
89 self notify(
"cancel_selection" );
95 self.pers[
"drone_strike_radar_used"] =
true;
96 self notify(
"cancel_selection");
100 self.pers[
"drone_strike_radar_used"] =
false;
106 self endon(
"emp_jammed" );
107 self endon(
"emp_grenaded" );
109 self waittill(
"confirm_location", location, yaw );
111 locationInfo = SpawnStruct();
112 locationInfo.origin = location;
113 locationInfo.yaw = yaw;
128 self AddWeaponStat( GetWeapon(
"drone_strike" ),
"used", 1 );
133 self thread
StartDroneStrike( location.origin, location.yaw, team, killstreak_id );
140 self util::waittill_any(
"disconnect",
"joined_team",
"joined_spectators",
"drone_strike_complete",
"emp_jammed" );
146 self endon(
"emp_jammed" );
147 self endon(
"joined_team" );
148 self endon(
"joined_spectators" );
149 self endon(
"disconnect" );
151 angles = ( 0, yaw, 0 );
152 direction = AnglesToForward( angles );
155 selectedPosition = ( position[0], position[1], height );
160 traceStartPos = ( position[0], position[1], height );
161 traceEndPos = ( position[0], position[1], -height );
162 trace = BulletTrace( traceStartPos, traceEndPos, 0, undefined );
163 targetPoint = ( (
trace[
"fraction" ] < 1.0 ) ?
trace[
"position" ] : ( position[0], position[1], 0.0 ) );
169 right = AnglesToRight( angles );
174 self thread
SpawnDrone( startPoint + rightOffset, forwardOffset + rightOffset, targetPoint, angles,
self.team, killstreak_id );
175 self thread
SpawnDrone( startPoint - rightOffset, forwardOffset - rightOffset, targetPoint, angles,
self.team, killstreak_id );
176 self thread
SpawnDrone( startPoint + leftOffset, forwardOffset + leftOffset, targetPoint, angles,
self.team, killstreak_id );
179 self playsound (
"mpl_thunder_flyover_wash");
183 self notify(
"drone_strike_complete" );
186 function SpawnDrone( startPoint, endPoint, targetPoint, angles, team, killstreak_id )
188 drone = SpawnPlane(
self,
"script_model", startPoint );
190 drone.targetname =
"drone_strike";
191 drone SetOwner(
self );
198 drone endon(
"delete" );
199 drone endon(
"death" );
201 drone.angles = angles;
206 PlayFxOnTag(
"killstreaks/fx_rolling_thunder_thruster_trails", drone,
"tag_fx");
215 weapon = GetWeapon(
"drone_strike" );
216 velocity = drone GetVelocity();
220 dZ = endPoint[2] - targetPoint[2];
221 dVxy = dXY * sqrt( halfGravity / dZ );
223 nvel = VectorNormalize( velocity );
224 launchVel = nvel * dVxy;
226 bomb =
self LaunchBomb( weapon, drone.origin, launchVel );
232 drone notify(
"hackertool_update_ent", bomb );
235 bomb.targetname =
"drone_strike";
236 bomb SetOwner(
self );
239 bomb playsound(
"mpl_thunder_incoming_start" );
256 drone SetCanDamage(
true );
259 drone.health = drone.maxhealth;
265 self endon(
"death" );
267 attacker =
self [[ level.figure_out_attacker ]]( attacker );
268 if( isdefined( attacker ) && ( !isdefined(
self.owner ) ||
self.owner
util::IsEnemyPlayer( attacker ) ) )
273 LUINotifyEvent( &
"player_callout", 2, &
"KILLSTREAK_DESTROYED_ROLLING_THUNDER_DRONE", attacker.entnum );
277 if( isdefined( params.ksExplosionFX ) )
278 PlayFXOnTag( params.ksExplosionFX,
self,
"tag_origin" );
280 self setModel(
"tag_origin" );
291 bomb endon(
"death" );
295 if( isdefined( isalive( bomb ) ) )
301 self endon(
"delete" );
302 self endon(
"death" );
304 self waittill(
"emp_deployed", attacker );
320 attacker endon(
"disconnect" );
321 attacker notify(
"DroneStrikeAwardScoreEvent_singleton" );
322 attacker endon(
"DroneStrikeAwardScoreEvent_singleton" );
325 attacker =
self [[ level.figure_out_attacker ]]( attacker );
329 LUINotifyEvent( &
"player_callout", 2, &
"KILLSTREAK_DESTROYED_ROLLING_THUNDER_ALL_DRONES", attacker.entnum );
337 if( isdefined(
self ) && isdefined( params.ksExplosionFX ) )
338 PlayFX( params.ksExplosionFX,
self.origin );
function processScoreEvent(event, player, victim, weapon)
#define DRONE_STRIKE_LOCATION_SELECTOR
function WaitForLocationSelection()
function EmpDamageDrone(attacker)
#define DRONE_STRIKE_LEFT_OFFSET
function ActivateDroneStrike()
function create_enemy_influencer(name, origin, team)
function SetupDamageHandling()
function DestroyDronePlane(attacker, weapon)
#define DRONE_STRIKE_END_OFFSET
function finishHardpointLocationUsage(location, usedCallback)
function getMinimumFlyHeight()
function play_killstreak_start_dialog(killstreakType, team, killstreakId)
function DroneStrikeLocationSelected(location)
function trace(from, to, target)
function addFlySwatterStat(weapon, aircraft)
#define DRONE_STRIKE_Z_OFFSET
function SelectDroneStrikePath()
function DroneStrikeAwardEMPScoreEvent(attacker, victim)
function get_low_health(killstreakType)
function MonitorDamage(killstreak_ref, max_health, destroyed_callback, low_health, low_health_callback, emp_damage, emp_callback, allow_bullet_damage)
function IsEnemyPlayer(player)
function WatchOwnerEvents(bomb)
function destroyedAircraft(attacker, weapon, playerControlled)
function isKillstreakAllowed(hardpointType, team)
function set_team_kill_penalty_scale(killstreakType, scale, isInventory)
function waittill_any(str_notify1, str_notify2, str_notify3, str_notify4, str_notify5)
#define DRONE_STRIKE_START_OFFSET
function get_max_health(killstreakType)
function register_dialog(killstreakType, informDialog, taacomDialogBundleKey, pilotDialogArrayKey, startDialogKey, enemyStartDialogKey, enemyStartMultipleDialogKey, hackedDialogKey, hackedStartDialogKey, requestDialogKey, threatDialogKey, isInventory)
#define INVALID_KILLSTREAK_ID
function SpawnDrone(startPoint, endPoint, targetPoint, angles, team, killstreak_id)
function WatchForEmp(owner)
function register_strings(killstreakType, receivedText, notUsableText, inboundText, inboundNearPlayerText, hackedText, utilizesAirspace=true, isInventory=false)
function killstreakStop(hardpointType, team, id)
#define DRONE_STRIKE_NAME
function killstreakStart(hardpointType, team, hacked, displayTeamMessage)
#define DRONE_STRIKE_COUNT
function set(str_field_name, n_value)
#define DRONE_STRIKE_MODEL
function configure_team(killstreakType, killstreakId, owner, influencerType, configureTeamPreFunction, configureTeamPostFunction, isHacked=false)
#define DRONE_STRIKE_FLIGHT_TIME
#define DRONE_STRIKE_RIGHT_OFFSET
#define DRONE_STRIKE_FORWARD_OFFSET
function StartDroneStrike(position, yaw, team, killstreak_id)
function play_taacom_dialog(dialogKey, killstreakType, killstreakId)
function enable_hacking(killstreakName, preHackFunction, postHackFunction)
function result(death, attacker, mod, weapon)
function BlowUpDroneStrike()
function WatchForKillstreakEnd(team, influencer, killstreak_id)
#define DRONE_STRIKE_SPAWN_INTERVAL
#define WAIT_SERVER_FRAME