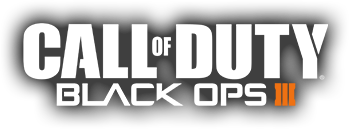 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\shared\system_shared;
2 #using scripts\shared\callbacks_shared;
3 #using scripts\shared\filter_shared;
4 #using scripts\shared\postfx_shared;
5 #insert scripts\shared\shared.gsh;
6 #using scripts\shared\util_shared;
8 #namespace water_surface;
10 #precache( "client_fx", "player/fx_plyr_water_jump_in_bubbles_1p" );
11 #precache( "client_fx", "player/fx_plyr_water_jump_out_splash_1p" );
13 #define WATER_DIVE_OVERLAY_TIME 0.7
14 #define WATER_SHEETING_OVERLAY_TIME 2.0
17 #define STOP_WATER_SHEETING { filter::disable_filter_water_sheeting( self, FILTER_INDEX_WATER_SHEET ); stop_player_fx( self ); }
20 #define STOP_WATER_DIVE { filter::disable_filter_water_dive( self, FILTER_INDEX_WATER_SHEET ); stop_player_fx( self );}
26 level._effect[
"water_player_jump_in"] =
"player/fx_plyr_water_jump_in_bubbles_1p";
27 level._effect[
"water_player_jump_out"] =
"player/fx_plyr_water_jump_out_splash_1p";
29 if ( isdefined( level.disableWaterSurfaceFX ) && level.disableWaterSurfaceFX ==
true )
39 if(
self != GetLocalPlayer( localClientNum ) )
42 if ( isdefined( level.disableWaterSurfaceFX ) && level.disableWaterSurfaceFX ==
true )
58 self notify(
"underwaterWatchBegin" );
59 self endon(
"underwaterWatchBegin" );
60 self endon(
"entityshutdown" );
64 self waittill(
"underwater_begin", teleported );
79 self notify(
"underwaterWatchEnd" );
80 self endon(
"underwaterWatchEnd" );
81 self endon(
"entityshutdown" );
85 self waittill(
"underwater_end", teleported );
100 self notify(
"water_surface_underwater_begin" );
101 self endon(
"water_surface_underwater_begin" );
102 self endon(
"entityshutdown" );
104 localClientNum =
self getlocalclientnumber();
108 if ( islocalclientdead( localClientNum ) ==
false )
110 self.firstperson_water_fx = PlayFXOnCamera( localClientNum, level._effect[
"water_player_jump_in"], (0,0,0), (1,0,0), (0,0,1) );
111 if ( !isdefined(
self.playingPostfxBundle ) ||
self.playingPostfxBundle !=
"pstfx_watertransition" )
113 self thread postfx::PlayPostfxBundle(
"pstfx_watertransition" );
120 self notify(
"water_surface_underwater_end" );
121 self endon(
"water_surface_underwater_end" );
122 self endon(
"entityshutdown" );
124 localClientNum =
self getlocalclientnumber();
125 if ( islocalclientdead( localClientNum ) ==
false )
127 if ( !isdefined(
self.playingPostfxBundle ) ||
self.playingPostfxBundle !=
"pstfx_water_t_out" )
129 self thread postfx::PlayPostfxBundle(
"pstfx_water_t_out" );
149 for ( i = 0; i < 0.05; i += 0.01 )
164 for ( i = 0.2; i > 0; i -= 0.01 )
174 for ( i = 0.2; i > 0; i -= 0.01 )
183 self notify(
"startWaterSheeting_singleton" );
184 self endon(
"startWaterSheeting_singleton" );
186 self endon(
"entityshutdown" );
201 rivulet1 = (i/2.0) - 0.19;
202 rivulet2 = (i/2.0) - 0.13;
203 rivulet3 = (i/2.0) - 0.07;
215 if ( IsDefined( localClient.firstperson_water_fx ) )
217 localClientNum = localClient getlocalclientnumber();
218 StopFx( localClientNum, localClient.firstperson_water_fx );
219 localClient.firstperson_water_fx = undefined;
function localplayer_spawned(localClientNum)
function on_localplayer_spawned(local_client_num)
function set_filter_water_dive_bubbles(player, filterid, amount)
#define FILTER_INDEX_WATER_SHEET
function startWaterDive()
function set_filter_water_sheet_speed(player, filterid, amount)
function init_filter_water_sheeting(player)
function enable_filter_water_dive(player, filterid)
#define WATER_SHEETING_OVERLAY_TIME
function set_filter_water_wash_color(player, filterid, red, green, blue)
function underwaterWatchBegin()
#define REGISTER_SYSTEM(__sys, __func_init_preload, __reqs)
function init_filter_water_dive(player)
#define STOP_WATER_SHEETING
function enable_filter_water_sheeting(player, filterid)
function set_filter_water_wash_reveal_dir(player, filterid, dir)
function underwaterEnd()
function underwaterWatchEnd()
function set_filter_water_sheet_reveal(player, filterid, amount)
function set_filter_water_scuba_dive_speed(player, filterid, amount)
function startWaterSheeting()
function underwaterBegin()
function set_filter_water_scuba_bubbles(player, filterid, amount)
function set_filter_water_scuba_bubble_attitude(player, filterid, amount)
function stop_player_fx(localClient)
function set_filter_water_sheet_rivulet_reveal(player, filterid, riv1, riv2, riv3)