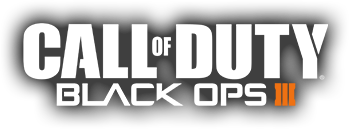 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\codescripts\struct;
3 #using scripts\shared\callbacks_shared;
4 #using scripts\shared\clientfield_shared;
5 #using scripts\shared\filter_shared;
6 #using scripts\shared\util_shared;
8 #using scripts\shared\abilities\_ability_player;
9 #using scripts\shared\abilities\_ability_power;
10 #using scripts\shared\abilities\_ability_util;
12 #insert scripts\shared\shared.gsh;
13 #insert scripts\shared\version.gsh;
15 #using scripts\shared\system_shared;
16 #using scripts\shared\visionset_mgr_shared;
17 #using scripts\shared\postfx_shared;
18 #using scripts\shared\lui_shared;
20 #insert scripts\shared\abilities\gadgets\_gadget_overdrive.gsh;
22 #define MAX_FLASH_ALPHA GetDvarFloat("scr_overdrive_flash_alpha", 0.7)
23 #define FLASH_FADE_IN_TIME GetDvarFloat("scr_overdrive_flash_fade_in_time", 0.075)
24 #define FLASH_FADE_OUT_TIME GetDvarFloat("scr_overdrive_flash_fade_out_time", 0.45)
25 #define OVERDRIVE_BOOST_FX_DURATION GetDvarFloat("scr_overdrive_boost_fx_time", 0.75)
26 #define OVERDRIVE_BLUR_AMOUNT GetDvarFloat( "scr_overdrive_amount", 0.15 )
27 #define OVERDRIVE_BLUR_INNER_RADIUS GetDvarFloat( "scr_overdrive_inner_radius", 0.6 )
28 #define OVERDRIVE_BLUR_OUTER_RADIUS GetDvarFloat( "scr_overdrive_outer_radius", 1 )
29 #define OVERDRIVE_BLUR_VELOCITY_SHOULDSCALE GetDvarInt( "scr_overdrive_velShouldScale", 1 )
30 #define OVERDRIVE_BLUR_VELOCITY_SCALE GetDvarInt( "scr_overdrive_velScale", 220 )
32 #define OVERDRIVE_SHOW_BOOST_SPEED_TOLERANCE GetDvarInt( "scr_overdrive_boost_speed_tol", 280 )
35 #precache( "client_fx", "player/fx_plyr_ability_screen_blur_overdrive" );
60 if(
self != GetLocalPlayer( localClientNum ) )
65 DisableSpeedBlur( localClientNum );
75 if ( !
self IsLocalPlayer() || IsSpectating( localClientNum,
false ) || ( (isdefined(level.localPlayers[localClientNum])) && (
self GetEntityNumber() != level.localPlayers[localClientNum] GetEntityNumber())) )
80 if( (newVal != oldval) && newval )
84 self UseAlternateAimParams();
88 else if ( (newVal != oldval) && !newval )
98 self notify(
"activation_flash");
99 self endon(
"activation_flash");
101 self endon(
"entityshutdown");
102 self endon(
"stop_player_fx");
103 self endon(
"disable_cybercom");
105 self.whiteFlashFade = 1;
109 self.whiteFlashFade = undefined;
115 if (isDefined(
self.firstperson_fx_overdrive))
117 StopFX(localClientNum,
self.firstperson_fx_overdrive);
118 self.firstperson_fx_overdrive = undefined;
120 self.firstperson_fx_overdrive = PlayFXOnCamera( localClientNum,
"player/fx_plyr_ability_screen_blur_overdrive", (0,0,0), (1,0,0), (0,0,1) );
126 self notify(
"watch_stop_player_fx");
127 self endon(
"watch_stop_player_fx");
128 self endon(
"entityshutdown");
132 if ( IsDefined( fx ) )
134 StopFx( localClientNum, fx );
135 self.firstperson_fx_overdrive = undefined;
142 self notify(
"stop_player_fx" );
153 self endon(
"overdrive_boost_fx_interrupt_handler");
154 self endon(
"end_overdrive_boost_fx");
155 self endon(
"entityshutdown");
166 if (isdefined(localClientNum))
169 self ClearAlternateAimParams();
171 DisableSpeedBlur( localClientNum );
172 self notify(
"end_overdrive_boost_fx");
180 self endon(
"disable_cybercom");
182 self endon(
"end_overdrive_boost_fx");
183 self endon(
"disconnect");
193 while (isDefined(
self))
196 v_player_velocity =
self GetVelocity();
197 v_player_forward = Anglestoforward(
self.angles);
198 n_dot = VectorDot(VectorNormalize(v_player_velocity), v_player_forward);
199 n_speed = Length(v_player_velocity);
204 if (!isdefined(
self.firstperson_fx_overdrive))
211 if (isdefined(
self.firstperson_fx_overdrive))
function init_filter_overdrive(player)
#define FILTER_INDEX_OVERDRIVE
#define OVERDRIVE_SHOW_BOOST_SPEED_TOLERANCE
function player_overdrive_handler(localClientNum, oldVal, newVal, bNewEnt, bInitialSnap, fieldName, bWasTimeJump)
function on_localclient_shutdown(func, obj)
#define CF_CALLBACK_ZERO_ON_NEW_ENT
function screen_fade(n_time, n_target_alpha=1, n_start_alpha=0, str_color="black", b_force_close_menu=false)
function on_localplayer_spawned(localClientNum)
#define OVERDRIVE_VISIONSET
#define FLASH_FADE_IN_TIME
function on_player_connect(local_client_num)
function on_localplayer_shutdown(localClientNum)
function watch_stop_player_fx(localClientNum, fx)
function register_visionset_info(name, version, lerp_step_count, visionset_from, visionset_to, visionset_type=VSMGR_VISIONSET_TYPE_NAKED)
function overdrive_boost_fx_interrupt_handler(localClientNum)
#define OVERDRIVE_VISIONSET_ALIAS
function overdrive_shutdown(localClientNum)
function stop_boost_camera_fx(localClientNum)
#define OVERDRIVE_BLUR_OUTER_RADIUS
#define OVERDRIVE_BLUR_VELOCITY_SHOULDSCALE
function on_localclient_connect(localClientNum)
#define OVERDRIVE_BOOST_FX_DURATION
#define FLASH_FADE_OUT_TIME
#define OVERDRIVE_BLUR_INNER_RADIUS
function waittill_any(str_notify1, str_notify2, str_notify3, str_notify4, str_notify5)
function activation_flash(localClientNum)
#define REGISTER_SYSTEM(__sys, __func_init_preload, __reqs)
#define OVERDRIVE_BLUR_AMOUNT
#define OVERDRIVE_BLUR_VELOCITY_SCALE
function enable_filter_overdrive(player, filterid)
function enable_boost_camera_fx(localClientNum)
function disable_filter_overdrive(player, filterid)
function boost_fx_on_velocity(localClientNum)
#define WAIT_CLIENT_FRAME
#define OVERDRIVE_VISIONSET_STEPS