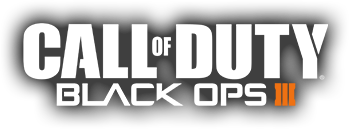 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\codescripts\struct;
3 #using scripts\shared\callbacks_shared;
4 #using scripts\shared\flagsys_shared;
5 #using scripts\shared\util_shared;
6 #using scripts\shared\vehicle_shared;
7 #using scripts\shared\vehicles\_escort_drone;
9 #using scripts\shared\abilities\_ability_player;
10 #using scripts\shared\abilities\_ability_power;
11 #using scripts\shared\abilities\_ability_util;
13 #insert scripts\shared\shared.gsh;
14 #insert scripts\shared\version.gsh;
15 #insert scripts\shared\abilities\_ability_util.gsh;
18 #using scripts\shared\system_shared;
20 #precache( "fx", "vehicle/fx_elec_teleport_escort_drone" );
39 level._effect[
"drone_spawn_fx"] =
"vehicle/fx_elec_teleport_escort_drone";
51 return self GadgetFlickering( slot );
86 self notify(
"gadget_drone_off" );
90 if ( IsDefined(
self.escort ) )
100 if ( IsDefined( time ) )
102 timeStr =
"^3" +
", time: " + time;
105 if ( GetDvarInt(
"scr_cpower_debug_prints" ) > 0 )
106 self IPrintlnBold(
"Gadget Drone:" + status + timeStr );
111 self endon(
"disconnect" );
118 eventTime =
self._gadgets_player[slot].gadget_flickertime;
124 if ( !
self GadgetFlickering( slot ) )
136 self endon(
"disconnect" );
137 self endon(
"gadget_drone_off" );
140 n_ahead_dist =
self._gadgets_player.escortLaunchDistance;
142 v_player_angles =
self GetPlayerAngles();
144 self.escort =
vehicle::spawn(
"veh_t7_drone_escort",
"ed",
"escort_drone", (0,0,0), v_player_angles );
145 self.escort.owner =
self;
146 self.escort.ignoreme =
true;
153 v_offset =
self GetPlayerViewHeight() + n_above_head;
155 self.escort.origin = (
self.origin[0],
self.origin[1],
self.origin[2] + v_offset );
157 self.escort.goal =
self.escort.origin + ( AnglesToForward( v_player_angles ) * n_ahead_dist );
159 if (
self.escort SetVehGoalPos(
self.escort.goal,
true, 2 ) )
170 PlayFX( level._effect[
"drone_spawn_fx"],
self.escort.origin );
172 self.escort Delete();
function register_gadget_is_flickering_callbacks(type, flickering_func)
function register_gadget_possession_callbacks(type, on_give, on_take)
function escort_drone_start_ai()
function gadget_drone_on_take(slot)
function gadget_drone_flicker(slot)
function clear(str_flag)
function register_gadget_is_inuse_callbacks(type, inuse_func)
function gadget_drone_on_flicker(slot)
function gadget_drone_off(slot)
function spawn(v_origin=(0, 0, 0), v_angles=(0, 0, 0))
function waittill_any_timeout(n_timeout, string1, string2, string3, string4, string5)
function get(kvp_value, kvp_key="targetname")
function register_gadget_flicker_callbacks(type, on_flicker)
function gadget_drone_is_inuse(slot)
function gadget_drone_on(slot)
function gadget_drone_spawn()
function gadget_drone_is_flickering(slot)
#define GADGET_TYPE_DRONE
function set_gadget_status(status, time)
function register_gadget_activation_callbacks(type, turn_on, turn_off)
function gadget_drone_on_connect()
#define REGISTER_SYSTEM(__sys, __func_init_preload, __reqs)
function drone_precache()
function gadget_drone_despawn()
function set(str_field_name, n_value)
function gadget_drone_on_give(slot, weapon)
function escort_drone_start_scripted()
function escort_drone_think(player)