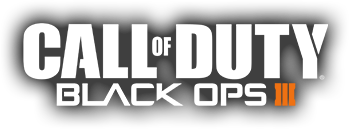 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\shared\ai\archetype_robot;
2 #using scripts\shared\ai\systems\ai_interface;
4 #insert scripts\shared\ai\archetype_robot.gsh;
6 #namespace RobotInterface;
93 "can_initiateaivsaimelee",
153 array(
"normal",
"gib_legs",
"remove_legs" ),
175 array(
"escort",
"guard",
"normal",
"marching",
"rambo",
"rusher",
"squadmember" ),
205 "phalanx_force_stance",
207 array(
"normal",
"stand",
"crouch" ) );
252 "rogue_allow_predestruct",
266 "rogue_allow_pregib",
289 array(
"level_0",
"level_1",
"forced_level_1",
"level_2",
"forced_level_2",
"level_3",
"forced_level_3" ),
302 "rogue_control_force_goal",
317 "rogue_control_speed",
319 array(
"walk",
"run",
"sprint" ),
333 "rogue_force_explosion",
378 "supports_super_sprint",
395 array(
"normal",
"procedural" ),
function robotTraversalAttributeCallback(entity, attribute, oldValue, value)
function rogueControlAttributeCallback(entity, attribute, oldValue, value)
function RegisterMatchedInterface(archetype, attribute, defaultValue, possibleValues, callbackFunction)
#define ROBOT_LIGHTS_DEATH
function RegisterRobotInterfaceAttributes()
#define ROBOT_LIGHTS_OFF
function robotMoveModeAttributeCallback(entity, attribute, oldValue, value)
#define ROBOT_LIGHTS_HACKED
#define ROBOT_LIGHTS_FLICKER
function rogueControlForceGoalAttributeCallback(entity, attribute, oldValue, value)
function RegisterVectorInterface(archetype, attribute, defaultValue, callbackFunction)
function rogueControlSpeedAttributeCallback(entity, attribute, oldValue, value)
function robotEquipMiniRaps(entity, attribute, oldValue, value)
function robotLights(entity, attribute, oldValue, value)
function robotForceCrawler(entity, attribute, oldValue, value)