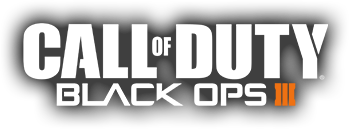 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\shared\ai\archetype_human;
2 #using scripts\shared\ai\systems\ai_interface;
4 #insert scripts\shared\archetype_shared\archetype_shared.gsh;
6 #namespace HumanInterface;
49 "can_initiateaivsaimelee",
112 array(
"normal",
"rambo" ),
128 "useAnimationOverride",
225 array(
"off",
"slow",
"fast" ),
function VignetteModeCallback(entity, attribute, oldValue, value)
function UseAnimationOverrideCallback(entity, attribute, oldValue, value)
function RegisterMatchedInterface(archetype, attribute, defaultValue, possibleValues, callbackFunction)
function cqbAttributeCallback(entity, attribute, oldValue, value)
function forceTacticalWalkCallback(entity, attribute, oldValue, value)
function moveModeAttributeCallback(entity, attribute, oldValue, value)
function RegisterHumanInterfaceAttributes()