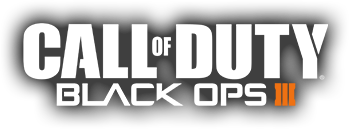 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\shared\array_shared;
2 #using scripts\shared\gameobjects_shared;
3 #using scripts\shared\math_shared;
4 #using scripts\shared\util_shared;
5 #using scripts\shared\weapons_shared;
6 #using scripts\shared\weapons\_weapon_utils;
7 #using scripts\shared\weapons\_weapons;
9 #insert scripts\shared\shared.gsh;
11 #using scripts\mp\_util;
12 #using scripts\mp\bots\_bot;
13 #using scripts\shared\bots\_bot;
14 #using scripts\shared\bots\_bot_combat;
15 #using scripts\shared\bots\bot_traversals;
16 #using scripts\shared\killstreaks_shared;
18 #insert scripts\mp\bots\_bot.gsh;
20 #define EXPLOSION_RADIUS_FRAG 256
21 #define EXPLOSION_RADIUS_FLASH 650
23 #define JUMP_TAG_RADIUS_SQ 16384 // 128 * 128
25 #namespace bot_combat;
56 if (
self IsReloading() ||
57 self IsSwitchingWeapons() ||
58 self IsThrowingGrenade() ||
60 self IsRemoteControlling() ||
62 self IsWeaponViewOnlyLinked() )
90 if( !IsDefined( level.dogtags ) )
95 if ( isdefined(
self.bot.goalTag ) )
100 self.bot.goalTag = undefined;
104 self BotSetGoal(
self.origin );
107 else if ( !
self.bot.goalTagOnGround &&
111 self BotSightTrace(
self.bot.goalTag ) )
116 else if ( !
self BotGoalSet() )
120 if ( isdefined( closestTag ) )
131 if ( !isdefined( enemy ) || IsPlayer( enemy ) )
136 killstreakType = undefined;
138 if ( isdefined( enemy.killstreakType ) )
140 killstreakType = enemy.killstreakType;
142 else if ( isdefined( enemy.parentStruct ) && isdefined( enemy.parentStruct.killstreakType ) )
144 killstreakType = enemy.parentStruct.killstreakType;
147 if ( !isdefined( killstreakType ) )
152 switch( killstreakType )
157 case "helicopter_gunner":
208 closestTag = undefined;
209 closestTagDistSq = undefined;
211 foreach( tag
in level.dogtags )
218 distSq = DistanceSquared(
self.origin, tag.origin );
220 if ( !isdefined( closestTag ) || distSq < closestTagDistSq )
223 closestTagDistSq = distSq;
232 self.bot.goalTag = tag;
234 traceStart = tag.origin;
235 traceEnd = tag.origin + (0,0,-64);
236 trace = BulletTrace( traceStart, traceEnd,
false, undefined );
238 self.bot.goalTagOnGround = (
trace[
"fraction"] < 1 );
function reload_weapon()
function mp_post_combat()
function path_to_trigger(trigger, radius)
function combat_toss_frag(origin)
function combat_tactical_insertion(origin)
function nearest_node(origin)
function threat_requires_launcher(enemy)
function combat_toss_flash(origin)
function trace(from, to, target)
function bot_pre_combat()
function use_killstreak()
function combat_throw_smoke(origin)
function get_closest_tag()
function set_goal_tag(tag)
function switch_weapon()
function can_interact_with(player)
function dot_product(origin)
#define JUMP_TAG_RADIUS_SQ
function combat_throw_proximity(origin)
function jump_to(target, vector)
function mp_pre_combat()
function combat_throw_tactical(origin)
function combat_throw_lethal(origin)
function bot_ignore_threat(entity)
function threat_switch_weapon()
function sprint_to_goal()