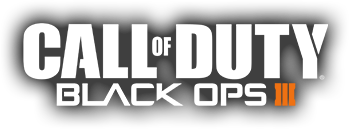 |
Black Ops 3 Source Code Explorer
0.1
An script explorer for Black Ops 3 by ZeRoY
|
Go to the documentation of this file. 1 #using scripts\codescripts\struct;
3 #using scripts\shared\audio_shared;
4 #using scripts\shared\array_shared;
5 #insert scripts\shared\shared.gsh;
37 level waittill (
"pl1");
48 if(isdefined(
self ) )
50 playsound(0,
"evt_light_start",
self.origin);
57 if(isdefined(
self ) )
60 playsound(0,
"evt_switch_progress",
self.origin);
61 playsound(0,
"evt_gen_start",
self.origin);
68 if(isdefined(
self ) )
70 playsound(0,
"evt_break_start",
self.origin);
77 if(isdefined(
self.script_noteworthy ) )
79 if(
self.script_noteworthy ==
"1" )
81 else if(
self.script_noteworthy ==
"2" )
83 else if(
self.script_noteworthy ==
"3" )
85 else if(
self.script_noteworthy ==
"4" )
87 else if(
self.script_noteworthy ==
"5" )
93 playsound(0,
"evt_switch_progress",
self.origin);
101 level waittill(
"pap1" );
102 homepad =
struct::get(
"homepad_power_looper",
"targetname" );
103 home_breaker =
struct::get(
"homepad_breaker",
"targetname" );
105 if(isdefined( homepad ))
109 if(isdefined( home_breaker ) )
121 level waittill(
"tp" + pad);
138 level waittill(
"tpw" + pad );
140 if(IsDefined(
self.script_sound ))
142 if(
self.targetname ==
"telepad_" + pad)
144 playsound( 0,
self.script_sound +
"_warmup",
self.origin );
146 playsound( 0,
self.script_sound +
"_cooldown",
self.origin );
148 if(
self.targetname ==
"homepad")
151 playsound( 0,
self.script_sound +
"_warmup",
self.origin );
152 playsound( 0,
self.script_sound +
"_cooldown",
self.origin );
185 level endon(
"scd" + pad );
189 level waittill(
"pac" + pad );
191 playsound( 0,
"evt_pa_buzz",
self.origin );
197 play = count == 20 || count == 15 || count <= 10;
200 playsound( 0,
"vox_pa_audio_link_" + count,
self.origin );
204 playsound( 0,
"evt_clock_tick_1sec", (0,0,0) );
208 playsound( 0,
"evt_pa_buzz",
self.origin );
217 level waittill(
"scd" + pad );
219 playsound( 0,
"evt_pa_buzz",
self.origin );
229 level waittill(
"tpc" + pad );
232 playsound( 0,
"evt_pa_buzz",
self.origin );
242 level waittill( location );
244 playsound( 0,
"evt_pa_buzz",
self.origin );
248 playsound( 0,
"evt_pa_buzz",
self.origin );
256 if( !IsDefined(
self.pa_is_speaking ) )
258 self.pa_is_speaking = 0;
261 if(
self.pa_is_speaking != 1 )
263 self.pa_is_speaking = 1;
264 self.pa_id = playsound( 0, alias,
self.origin );
265 while( SoundPlaying(
self.pa_id ) )
269 self.pa_is_speaking = 0;
277 level waittill(
"t2d" );
278 playsound( 0,
"evt_teleport_2d_fnt", (0,0,0) );
279 playsound( 0,
"evt_teleport_2d_rear", (0,0,0) );
285 level waittill (
"pl1");
286 playsound( 0,
"evt_power_up_2d", (0,0,0) );
291 level waittill(
"pap1" );
292 playsound( 0,
"evt_linkall_2d", (0,0,0) );
301 level waittill (
"pl1");
303 playsound( 0,
"evt_pa_buzz",
self.origin );
310 level waittill (
"pl1");
311 playsound( 0,
"evt_crazy_power_left", (-510, 394, 102) );
312 playsound( 0,
"evt_crazy_power_right", (554, -1696, 156) );
317 level waittill (
"pl1");
318 playsound( 0,
"evt_flip_sparks_left", (511, -1771, 116 ) );
319 playsound( 0,
"evt_flip_sparks_right", (550, -1771, 116 ) );
338 playsound( 0,
"amb_creepy_whispers", (-339, 271, 207));
339 playsound( 0,
"amb_creepy_whispers", (234, 110, 310));
340 playsound( 0,
"amb_creepy_whispers", (-17, -564, 255));
341 playsound( 0,
"amb_creepy_whispers", (743, -1859, 210));
342 playsound( 0,
"amb_creepy_whispers", (790, -748, 181));
343 wait RandomIntRange(1,4);
351 playsound( 0,
"amb_creepy_children", (-2637, -2403, 413));
function pa_teleport(pad)
function pa_level_start()
function play_backwards_children()
function play_added_ambience()
function power_audio_2d()
function generator_sound()
function play(animation, v_origin_or_ent, v_angles_or_tag, n_rate=1, n_blend_in=.2, n_blend_out=.2, n_lerp, b_link=false)
function get_array(kvp_value, kvp_key="targetname")
function pa_electric_trap(location)
function pa_single_init()
function get(kvp_value, kvp_key="targetname")
function teleport_pad_init(pad)
function pa_countdown_success(pad)
function breakers_sound()
function pa_countdown(pad)
function play_flux_whispers()
function switch_progress_sound()
function teleportation_audio(pad)
function pa_play_dialog(alias)
function playloopat(aliasname, origin)